Explore the ins and outs of embedding images using Base64 encoding and the img
element’s src
attribute. Learn about the advantages and drawbacks of employing Base64 encoding for image embedding within HTML documents. Additionally, discover a step-by-step guide on how to seamlessly insert Base64-encoded images into your web content using the img src
attribute. This comprehensive guide equips you with the knowledge to effectively leverage Base64 encoding for image embedding in your web development endeavors.
Benefits of Using Base64 for Image Embedding
Base64 encoding offers several advantages when it comes to embedding images in web pages:
- Reduced HTTP Requests: By using Base64 to embed images directly into HTML, you can avoid making additional HTTP requests to fetch image files.
- Portability: Base64-encoded images are portable because they are self-contained within the HTML document, which makes it simpler to relocate or distribute the information without worrying about shattered image links.
- Simplified Deployment: Base64-encoded images make deployment and maintenance easier because you don’t have to manage distinct image files on your server.
Disadvantages of Using Base64 for Image Embedding
While Base64 encoding has a number of advantages for embedding images, there are also disadvantages to take into account:
- Increased HTML Size: Base64-encoded images can add a lot of space to your HTML files, which may cause them to load more slowly, especially for larger images.
- No Caching Benefits: Base64-encoded images cannot be separately cached, unlike distinct image files, hence there are no caching advantages. The complete HTML file must be reloaded whenever an image is changed, which may reduce the effectiveness of caching.
- Encoding overhead: Base64 encoding increases the size of the encoded image relative to the binary version of the image by adding additional characters to represent binary data.
- Compatibility: While the majority of contemporary browsers can display photos that have been Base64-encoded, some older browsers or email clients might be unable to do so.
- Maintenance: Updating or altering images while utilizing Base64 encoding is more difficult because new HTML code must be written.
What is img src in HTML?
The img element in HTML is used for embedding images into websites. The source URL of the image file that should be shown on the webpage is specified by the src property of the img element. This URL often directs users to an external picture file that is stored on a server. The image is shown on the page by the browser after being fetched from the specified URL.
However, you may instead use Base64 encoding to embed the image directly within the src property rather of connecting to an external image file. This is accomplished by immediately inserting the Base64-encoded picture data into the src attribute value, so doing away with the requirement for an additional image file.
What is Data URI?
When embedding resources like images, audio files, or even full documents within HTML or CSS files, a Data URI (Uniform Resource Identifier) is a technique that enables data to be put directly in the URL. A Data URI explicitly encodes the data from the external resource into the URL itself rather than referencing it via a conventional URL.
It’s crucial to prefix the Base64 code with a Data URI scheme before embedding Base64-encoded pictures within the src property of the img element. The “data:image/” coding scheme notifies the browser that the content after it is an inline data source and provides the image format information. The Base64-encoded image data must come before the Data URI to enable appropriate rendering and presentation of the embedded picture on the webpage.
How to Embed Images in img src With Base64 Encoding?
It is simple to include images using Base64 encoding in the img element’s src attribute. The Base64-encoded picture data is directly included into the property value rather than being referenced by an external image file. Here’s an example:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg==">
The src attribute of the img element in the example above begins with “data:image/png;base64,” and then the Base64-encoded image data. After the” image/” portion, the image format (in this case, PNG) is provided. Using this method, you can easily integrate photos into your HTML, eliminating the need for additional image files and improving online performance.
How to Convert Images to Base64
Numerous approaches are at your disposal when it comes to transforming images into Base64 format. One commonly employed method involves leveraging online converters dedicated to image-to-Base64 conversion, widely accessible across various platforms. Another avenue is through the utilization of our website’s complimentary online tool, specially designed for seamless Base64 conversion.
For those seeking a more hands-on approach, the option exists to carry out the conversion through bespoke code. This process involves delving into the image file, employing Base64 to encode its binary data, and subsequently extracting the resultant encoded Base64 string.
Example of Converting Images to Base64 in JavaScript
Converting images to Base64 encoding is a typical process in web development, and it is frequently used for scenarios such as embedding images straight into HTML. Let’s look at a simple JavaScript sample to see how this process works.
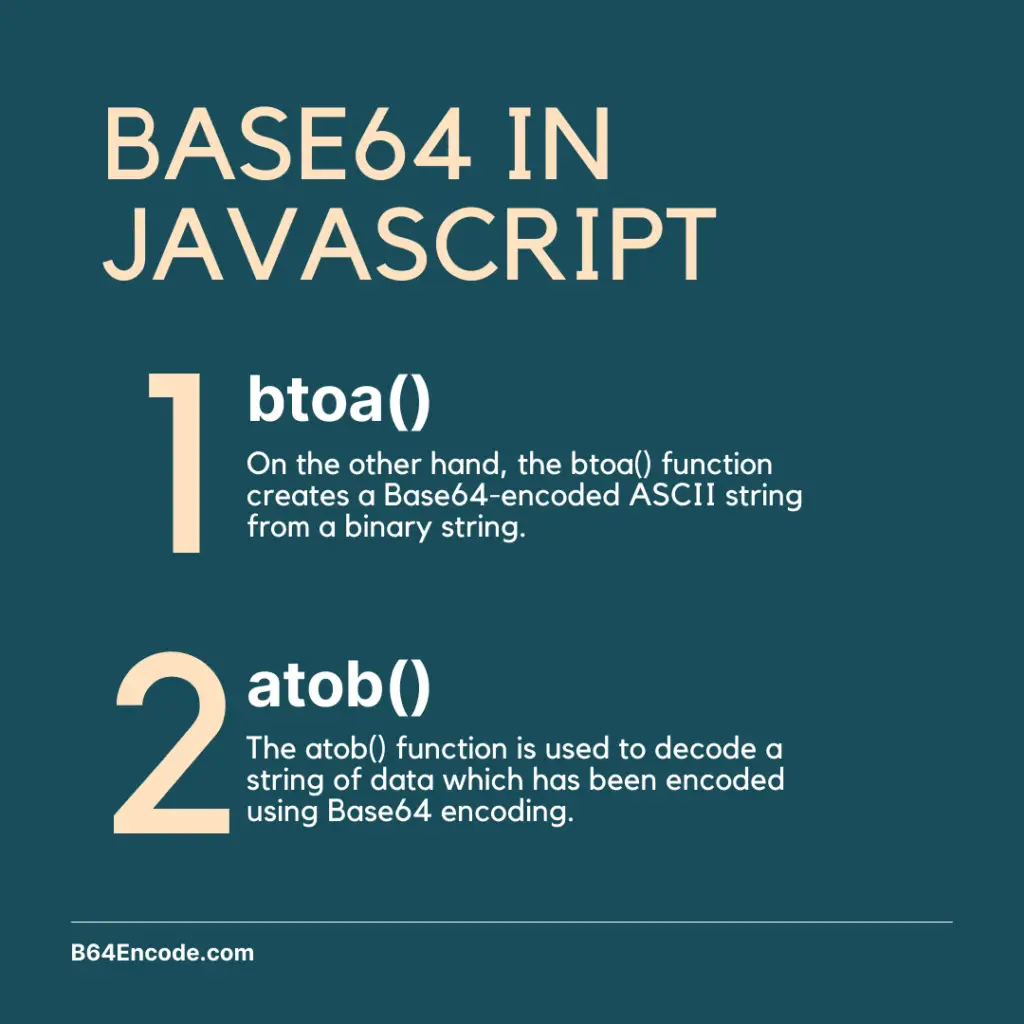
Assume you have a “image.png” picture file that you want to convert to Base64 format. Here’s how you do it with the FileReader API and the btoa() function:
// Select the input element where the user uploads the file const input = document.querySelector('input[type="file"]'); // Listen for the 'change' event when a file is selected input.addEventListener('change', (event) => { const file = event.target.files[0]; // Use FileReader to read the file as a Data URL const reader = new FileReader(); reader.onload = (readerEvent) => { const dataURL = readerEvent.target.result; // Remove the "data:image/png;base64," prefix const base64Data = dataURL.split(',')[1]; // Encode the Base64 data const encodedData = btoa(base64Data); // Now 'encodedData' contains the Base64-encoded file data }; // Read the file as a Data URL reader.readAsDataURL(file); });
Here is what happens in the code above:
- The
input
variable is assigned the result of callingdocument.querySelector()
with the argument'input[type="file"]'
. This selects the<input type="file">
element on the page. - When the user picks a file, an event listener is attached to the input element to listen for the ‘change’ event.
- When the
'change'
event is triggered, the first file in theevent.target.files
array is assigned to thefile
variable. - The reader variable is assigned a new FileReader object that is created.
- An event listener is added to the
reader
object to listen for the'load'
event, which is triggered when the file has been read as a Data URL. - When the
'load'
event is triggered, the result of reading the file as a Data URL is assigned to thedataURL
variable. - The
"data:image/png;base64,"
prefix is removed from thedataURL
by calling.split(',')[1]
, and the result is assigned to thebase64Data
variable. - The
base64Data
variable is then passed as an argument to thebtoa()
function, which encodes it in Base64 and returns the result. This result is assigned to theencodedData
variable. - Finally, the
reader.readAsDataURL()
method is called with thefile
variable as an argument, which starts reading the file as a Data URL.