Base64 encoding is a popular method for representing binary data in a readable and ASCII-compatible format. It’s widely used for a variety of purposes, including data transmission. In this article, we’ll look at Base64 encoding and decoding in Python.
What is Base64?
Base64 is a binary-to-text encoding method that is used to encode binary data into a text-based format, such as photos, audio files, or other digital data. It is intended to represent binary data in a format that is acceptable for text-based protocols like email or HTTP, where particular characters may be interpreted differently or get corrupted if they directly represent binary data.
Every three bytes of binary data are represented as four letters in the Base64 alphabet in Base64 encoding. This makes the encoded data longer than the original binary data, but it assures that it only contains ASCII characters, making it safe for transmission over text-based protocols.
What is Python?
Python is a high-level, general-purpose programming language known for its readability and simplicity. It was created by Guido van Rossum and first released in 1991. Python has become one of the most popular programming languages, widely used in various domains, including web development, data science, artificial intelligence, scientific computing, automation, and more.
Python has built-in support for Base64 encoding and decoding through the base64
module. This module provides functions for encoding binary data to Base64 and decoding Base64-encoded data back to binary.
How to Import Base64 in Python?
It is simple to import and use the base64
module in Python. Using the import
statement, you can import the module and then use the various functions it provides for Base64 encoding and decoding.
Here’s how you can import the base64
module and use its functions:
import base64
Base64 Encoding in Python
The base64 module provides a comprehensive set of functions for encoding and decoding data using the Base64 algorithm. The module’s ease of use and efficiency make it a popular tool for handling various data conversion tasks.
The base64
module in Python includes two main functions, the b64encode() function accepts binary data and returns the Base64-encoded version as bytes.
The module also includes the urlsafe_b64encode() function, which produces Base64 encoded output suitable for use in URLs and file names where certain characters may require special handling.
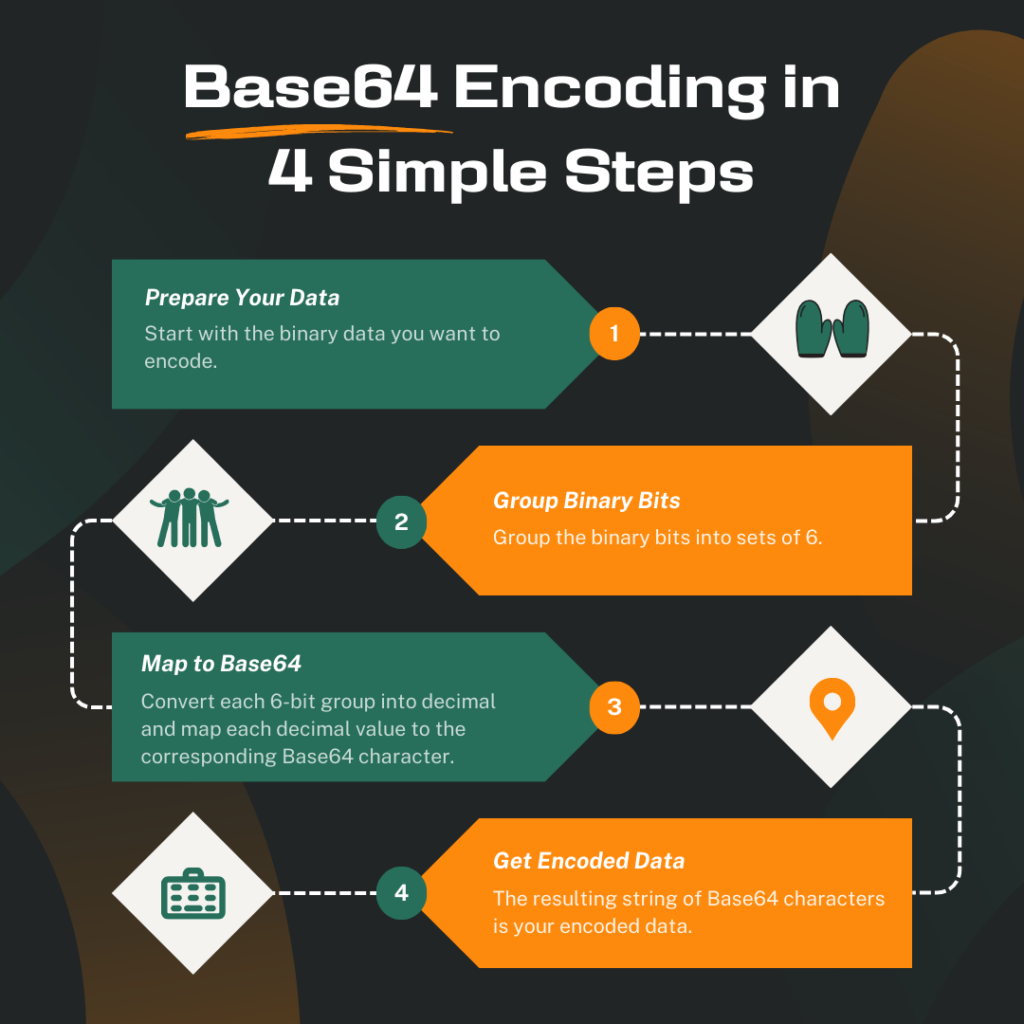
Example of Base64 Encoding in Python
Now let’s take a look at an example to show Base64 encoding in Python.
import base64 # Binary data to be encoded data_to_encode = b"B64Encode.com" # Encoding the data encoded_bytes = base64.b64encode(data_to_encode) encoded_string = encoded_bytes.decode('utf-8') print("Original Data:", data_to_encode) print("Encoded Data:", encoded_string)
In this example, we import the base64
module and then provide the binary data (data_to_encode
) that we want to encode using Base64. We use the b64encode()
function to perform the encoding, and then decode the resulting bytes into a string using the utf-8
encoding. Finally, we print both the original data and the Base64-encoded data.
It’s important to note that the b64encode()
function returns bytes, which is why we decode it to a string for printing purposes. Furthermore, the Base64 encoding output will only contain characters from the Base64 character set, making it secure for transmission and storage.
Base64 Decoding in Python
Python’s base64 module also includes a function for decoding data encoded with the Base64 algorithm. The function b64decode() accepts Base64-encoded data and returns the original binary data as bytes.
The module includes, in addition to the standard b64decode() function, the urlsafe_b64decode() function, which can be used to decode data encoded with the urlsafe_b64encode() function. This function is useful for decoding data contained in URLs or file names where certain characters may require special treatment.
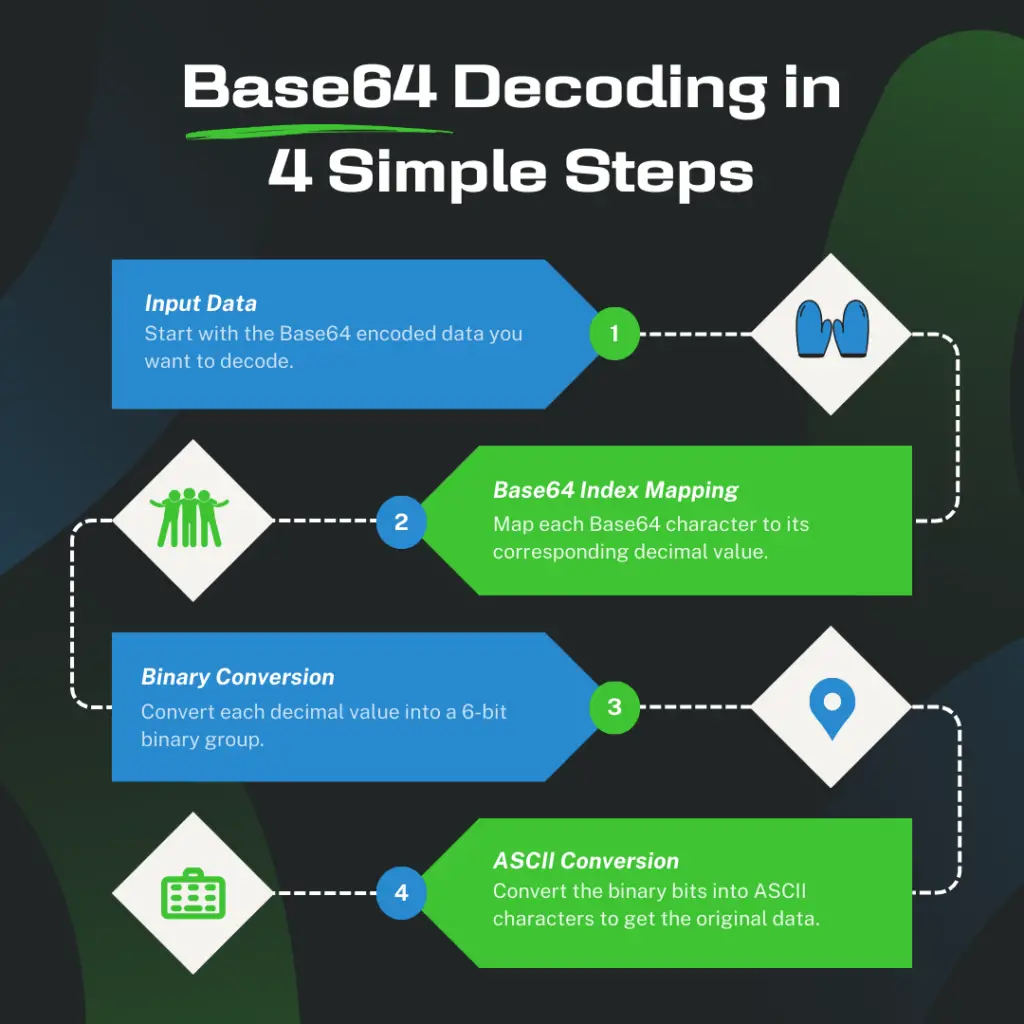
Example of Base64 Decoding in Python
This example shows you how to use the base64 module in Python to decode base64:
import base64 # Base64-encoded data encoded_data = "QjY0RW5jb2RlLmNvbQ==" # Decoding the data decoded_bytes = base64.b64decode(encoded_data) decoded_string = decoded_bytes.decode('utf-8') print("Encoded Data:", encoded_data) print("Decoded Data:", decoded_string)
First we import the base64
module, then we declare a Base64-encoded string (encoded_data
). We have to use the b64decode()
function to decode the Base64-encoded data. The resulting bytes are then decoded into a string using the utf-8
encoding. Finally, we print both the original encoded data and the decoded data.