This article looks into the essential Java programming language procedures of Base64 encoding and decoding. It discusses the significance of these operations and their implementation in Java as a concise yet adaptable guide. This article serves as a guide for developers looking to grasp Base64 encoding and decoding in Java, covering everything from built-in capabilities to practical applications.
What is Base64?
Base64 is like a special translator that takes binary data and turns it into a string of letters, numbers, and symbols that computers can easily handle. This allows us to send information like images or files across systems that can only deal with text.
Think of it like packing your suitcase for a trip. Base64 takes the binary data (your clothes) and folds it neatly into a format that fits perfectly within the suitcase (the text-based system). Sometimes, there might be a little extra space left over, so Base64 uses padding characters like ‘=’ to fill it up, ensuring everything is secure and organized.
While Base64 makes the data a bit bigger, it’s incredibly reliable and works perfectly in text-based environments like the internet. That’s why it’s so popular for sending images, files, and other binary data across websites and applications.
While Base64 encoding does not provide encryption or security in and of itself, it is a necessary tool for operations such as transferring binary data over URLs, embedding graphics within web pages, and attaching files to emails. Because of its ubiquitous support across programming languages and ease of usage, it is a beneficial method in a wide range of programming applications.
What is Java?
Java is a popular high-level programming language that is well-known for its platform freedom, object-oriented design, and large standard library. Java, developed by Sun Microsystems (bought by Oracle Corporation), was first launched in 1995 and has since grown in popularity due to its adaptability and applicability for a wide range of software development applications.
Key Features of Java:
- Platform Independence: Java’s core design philosophy revolves around platform independence, embodied in the “write once, run anywhere” (WORA) paradigm. This enables developers to create applications using a single codebase that can execute seamlessly across various operating systems and hardware architectures. This remarkable feat is achieved through the crucial role of the Java Virtual Machine (JVM).
- Object-Oriented: Java’s core design philosophy is deeply rooted in the principles of object-oriented programming (OOP). This paradigm fosters a structured and modular approach to software development by leveraging the concepts of classes and objects. Classes serve as blueprints that define the characteristics and behaviors of specific entities within the program. Objects, instantiated from these classes, encapsulate both data (attributes) and the actions they can perform (methods).
- Strongly Typed: Java adheres to a static typing paradigm, requiring explicit declaration of variable types during development. This means that the data type associated with each variable must be specified at the time of declaration.
- Rich Standard Library: Java boasts a comprehensive and robust Standard Library, also known as the Java Class Library (JCL). This library serves as a rich repository of pre-built classes and functions that developers can readily leverage in their projects. The JCL offers a vast array of functionalities, encompassing: File i/O, Networking, Data Manipulation, Collections Framework, Security, Concurrency.
- Memory Management: Java provides automatic memory management via the garbage collection process. This assists developers in avoiding memory leaks and efficiently managing memory.
- Security: Java includes security measures to prevent unwanted access and ensure secure code execution. The “sandbox” environment of the platform limits potentially dangerous acts.
Does Java Support Base64?
Yes, Java provides comprehensive support for Base64 encoding and decoding through its standard library. This support is offered in the java.util
package, specifically the java.util.Base64
class. This class was introduced in Java 8 and has become the standard way to handle Base64 encoding and decoding tasks.
The java.util.Base64
class offers two types of encoders and decoders:
- Basic Encoder/Decoder: This provides RFC 4648-compliant Base64 encoding and decoding. It uses a set of 64 distinct characters to encode binary data into ASCII characters (A-Z, a-z, 0-9, and ‘+’ and ‘/’). To maintain interoperability with email systems, the basic encoder inserts line breaks every 76 characters.
- URL Encoder/Decoder: This variant of Base64 encoding substitutes the basic encoder’s ‘+’ and ‘/’ characters with ‘-‘ and ‘_’, respectively. It’s frequently used in URLs to avoid problems with web servers misinterpreting characters as special characters.
The java.util.Base64
class includes methods for encoding and decoding data using both the basic and URL-safe encoders/decoders. These methods accept byte[]
arrays and return Base64-encoded or decoded byte[]
arrays, making it convenient for working with binary data.
Base64 Encoding in Java
The java.util.Base64
class, introduced with the release of Java 8, provides extensive support for Base64 encoding and decoding. In comparison to prior Java versions, this class provides a more versatile and efficient approach to work with Base64-encoded data.
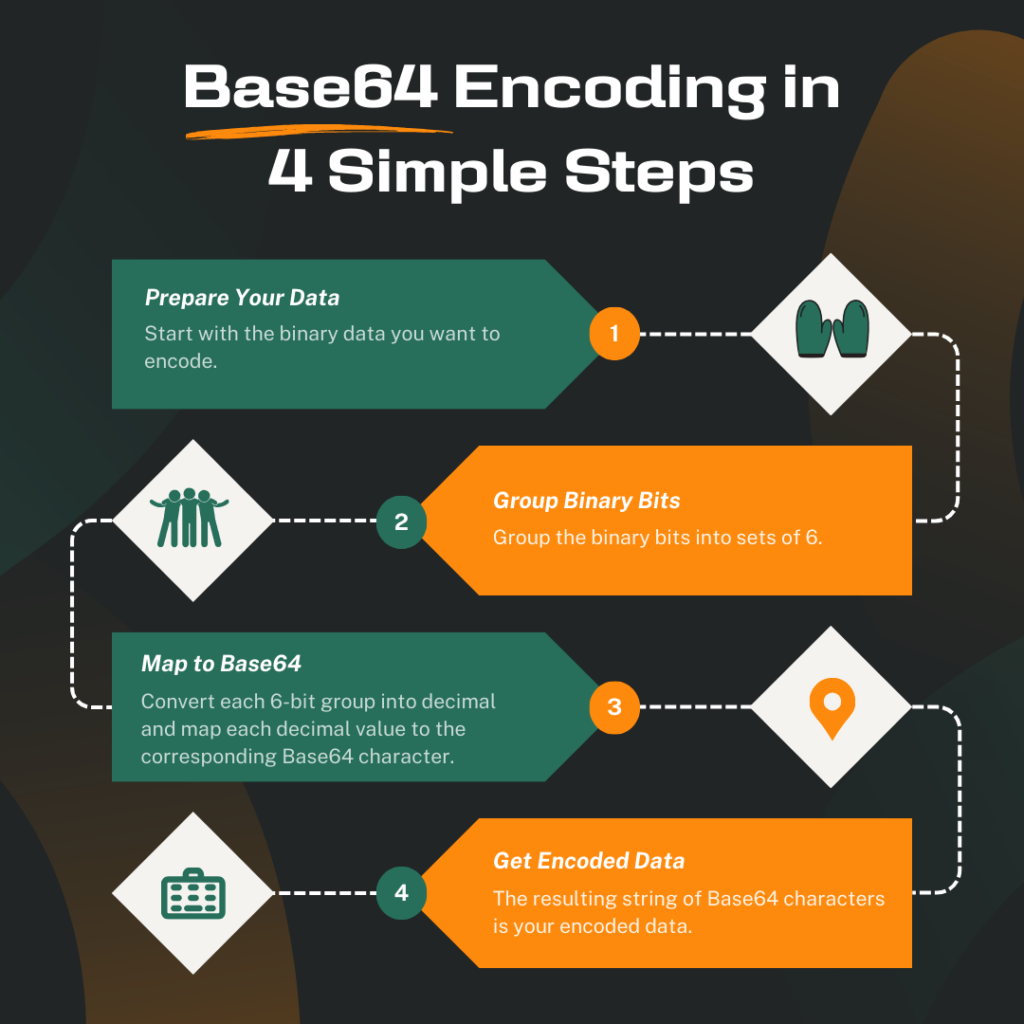
Base64 Encoding in Java 8 and Newer Versions
With the release of Java 8, the language introduced the java.util.Base64
class, which provides comprehensive support for Base64 encoding and decoding. This class offers a more flexible and efficient way to work with Base64-encoded data compared to previous versions of Java.
The java.util.Base64
class provides two different types of encoding: basic and URL-safe. Both methods allow you to encode binary data into Base64-encoded strings.
In the next chapter we will look at the next two methods:
- Basic Encoding: java.util.Base64.Encoder
- URL-Safe Encoding: java.util.Base64.getUrlEncoder()
Example: Base64 Encoding in Java
The basic encoding method produces a Base64-encoded string with standard Base64 characters (A-Z, a-z, 0-9, +, /). To use basic encoding, you first obtain an instance of the Base64.Encoder
class and then call the encodeToString
method.
import java.util.Base64; public class Base64Example { public static void main(String[] args) { byte[] binaryData = "B64Encode".getBytes(); Base64.Encoder encoder = Base64.getEncoder(); String base64String = encoder.encodeToString(binaryData); System.out.println("Basic Encoding: " + base64String); } }
The URL-safe encoding method produces a Base64-encoded string where the characters ‘+’ and ‘/’ are replaced with ‘-‘ and ‘_’. This is useful when encoding data for URLs. To use URL-safe encoding, you can directly obtain an instance of the URL-safe encoder and call the encodeToString
method.
import java.util.Base64; public class Base64Example { public static void main(String[] args) { byte[] binaryData = "B64Encode".getBytes(); Base64.Encoder urlEncoder = Base64.getUrlEncoder(); String urlSafeString = urlEncoder.encodeToString(binaryData); System.out.println("URL-Safe Encoding: " + urlSafeString); } }
It is vital to note that the Base64.Encoder instances are not thread-safe and are stateful. If you intend to utilize them in several threads, you should create a new encoder instance for each thread.
Example: PDF to Base64 in Java
Converting a PDF file to a Base64-encoded string can be beneficial in situations when the PDF material needs to be embedded directly into a document, delivered over a network, or stored as a text-based form. In this example, we’ll show how to do so with Java’s java.util.Base64
class.
Assume you have a PDF file named “b64encode.pdf” that you’d like to encode. How to read a PDF file and convert it to a Base64-encoded string:
import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.Base64; public class PDFToBase64Example { public static void main(String[] args) { String filePath = "path/to/b64encode.pdf"; try { // Read the PDF file as bytes Path path = Paths.get(filePath); byte[] pdfBytes = Files.readAllBytes(path); // Encode the PDF bytes using Base64 Base64.Encoder encoder = Base64.getEncoder(); String base64PDF = encoder.encodeToString(pdfBytes); System.out.println("PDF to Base64 Encoding:"); System.out.println(base64PDF); } catch (IOException e) { System.err.println("An error occurred: " + e.getMessage()); } } }
In this example:
- We specify the path to the PDF file to be encoded.
- To read the content of the PDF file as an array of bytes, we utilize the Files.readAllBytes method.
- Using Base64, we obtain an instance of the Base64.Encoder class.getEncoder().
- To turn the PDF bytes into a Base64-encoded string, we use the encodeToString function on the encoder instance.
- Finally, the Base64-encoded string is printed to the console.
Base64 Decoding in Java
Base64 decoding is the process of converting a Base64-encoded string back into its original binary format. Java provides built-in support for Base64 decoding through the java.util.Base64
class. This functionality allows you to reverse the process of Base64 encoding and retrieve the original data from its textual representation. To perform Base64 decoding in Java, you can use the Base64.Decoder
class provided by the java.util.Base64
package.
In Java, the java.util.Base64
class provides support for both basic and URL-safe decoding. The Base64
class has a unified decoding interface through the Decoder
interface, but it offers two specialized implementations: Decoder
for basic decoding and UrlDecoder
for URL-safe decoding.
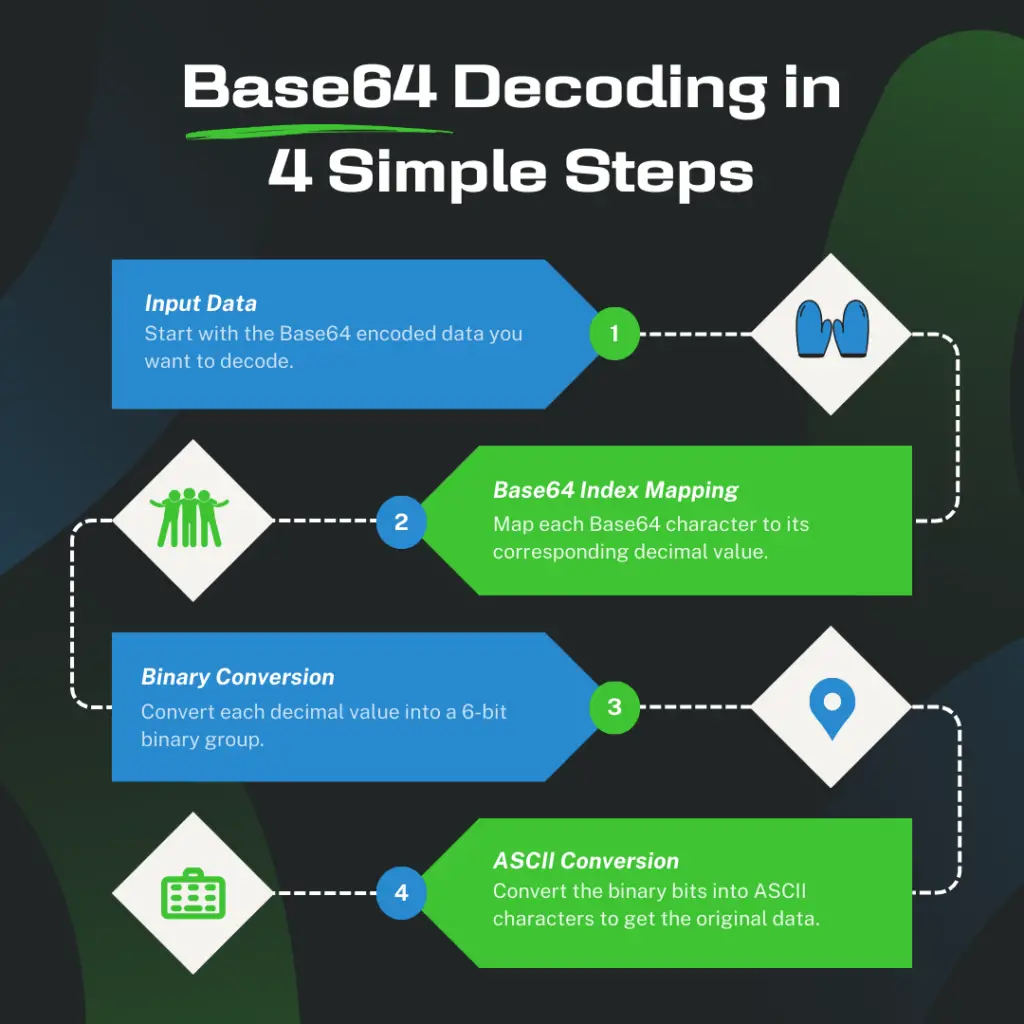
Example: Base64 Decoding in Java
In the first example, we use the Basic Decoder by obtaining a Decoder
instance with Base64.getDecoder()
. The decode()
method is then used to decode the Base64-encoded data.
import java.util.Base64; public class BasicDecoderExample { public static void main(String[] args) { String base64EncodedData = "QjY0RW5jb2Rl"; // Sample Base64-encoded data // Using the Basic Decoder Base64.Decoder basicDecoder = Base64.getDecoder(); byte[] decodedBytes = basicDecoder.decode(base64EncodedData); String decodedString = new String(decodedBytes); System.out.println("Decoded String (Basic Decoder): " + decodedString); } }
In the second example, we use the URL-Safe Decoder by obtaining a Decoder
instance with Base64.getUrlDecoder()
. The URL-Safe Decoder replaces the ‘+’ and ‘/’ characters with ‘-‘ and ‘_’ respectively, making it suitable for use in URLs and filenames.
import java.util.Base64; public class URLSafeDecoderExample { public static void main(String[] args) { String urlSafeEncodedData = "QjY0RW5jb2Rl"; // Sample URL-safe Base64-encoded data // Using the URL-Safe Decoder Base64.Decoder urlSafeDecoder = Base64.getUrlDecoder(); byte[] decodedBytes = urlSafeDecoder.decode(urlSafeEncodedData); String decodedString = new String(decodedBytes); System.out.println("Decoded String (URL-Safe Decoder): " + decodedString); } }
Example: Base64 to PDF in Java
import java.io.FileOutputStream; import java.io.IOException; import java.util.Base64; public class Base64ToPDFExample { public static void main(String[] args) { // Sample Base64-encoded PDF data String base64EncodedPDFData = "QjY0RW5jb2Rl"; // Decode Base64-encoded PDF data Base64.Decoder base64Decoder = Base64.getDecoder(); byte[] pdfBytes = base64Decoder.decode(base64EncodedPDFData); // Write the decoded PDF bytes to a file try (FileOutputStream fos = new FileOutputStream("decoded.pdf")) { fos.write(pdfBytes); System.out.println("PDF file successfully created."); } catch (IOException e) { System.err.println("An error occurred while creating the PDF file: " + e.getMessage()); } } }
We begin with a Base64-encoded PDF data string in this example. The Base64 class’s Basic Decoder is then used to decode the Base64-encoded data into a byte array representing the PDF content.
The decoded PDF bytes are then written to a new PDF file called “decoded.pdf” using a FileOutputStream. After use, the try block with the FileOutputStream will immediately shut the stream.
If the decoding and file writing processes are successful, a message indicating successful PDF file creation will be printed. An error notice will be presented if any errors occur during the process.
Base64 in Java 7
Until the java.util.Base64 class was introduced in Java 8, developers had to rely on external libraries or manual implementations to handle Base64 encoding and decoding in Java. However, Java 7 added limited Base64 support via the javax.xml.bind.DatatypeConverter class.
The DatatypeConverter
class provides methods to convert binary data to and from Base64-encoded strings. While not as feature-rich as the java.util.Base64
class, it can be used in Java 7 environments for basic encoding and decoding.
Here’s a brief overview of how to use DatatypeConverter
for Base64 encoding and decoding:
Encoding Binary Data to Base64 in Java 7
To encode binary data to a Base64-encoded string using DatatypeConverter
, you can use the printBase64Binary
method. This method accepts a byte[]
array and returns the corresponding Base64-encoded string.
import javax.xml.bind.DatatypeConverter; public class Base64Example { public static void main(String[] args) { byte[] binaryData = "B64Encode".getBytes(); String base64String = DatatypeConverter.printBase64Binary(binaryData); System.out.println("Base64 Encoded: " + base64String); } }
Decoding Base64 to Binary Data in Java 7
To decode a Base64-encoded string back to its original binary form, you can use the parseBase64Binary
method. This method accepts a Base64-encoded string and returns the corresponding byte[]
array.
import javax.xml.bind.DatatypeConverter; public class Base64Example { public static void main(String[] args) { String base64String = "QjY0RW5jb2Rl"; byte[] decodedData = DatatypeConverter.parseBase64Binary(base64String); String originalString = new String(decodedData); System.out.println("Decoded: " + originalString); } }