Base64 encoding and decoding are core web development techniques that play an important role in handling binary data within textual settings. PHP, as a robust and widely used scripting language, includes support for these actions by default. In this post, we’ll look at Base64 encoding and decoding in PHP, and how they help developers manipulate binary data easily within their applications.
What is Base64?
Base64 is a binary-to-text encoding technique designed to transform binary data into a printable ASCII string format. This method is extensively employed to represent data in a manner that facilitates secure transmission across text-based protocols. The encoding process enables the efficient conveyance of binary data, such as images or files, by converting it into a format comprised of easily readable text characters.
What is PHP?
PHP (Hypertext Preprocessor) is a popular open-source scripting language intended primarily for web development. It’s embedded in HTML and runs on the server, allowing developers to create dynamic and interactive online applications. PHP scripts may generate dynamic content, manage databases, manipulate files, and perform a variety of other server-side functions. PHP has been one of the most popular programming languages for web development since its creation in the mid-1990s, owing to its ease of use, versatility, and huge community support.
Key Features of PHP:
- Open Source: PHP is open source, which means it is available for free and has a big developer community contributing to its growth and improvement.
- Cross-Platform: PHP operates on a variety of platforms, including Windows, macOS, Linux, and many others.
- Server-Side Scripting: PHP scripts are processed on the server before being delivered to the client’s browser, enabling dynamic content generation and interactivity.
- Integration: PHP is easily connected with databases such as MySQL, making it a powerful tool for data-driven online applications.
- Embedding: PHP code can be embedded within HTML, allowing developers to mix server-side functionality with client-side markup in a seamless manner.
- Extensive Library Support: PHP comes with a plethora of libraries, frameworks, and extensions that help to simplify and accelerate development.
Does PHP Support Base64?
Yes, PHP includes functions and capabilities for Base64 encoding and decoding. Base64 encoding is a typical web development approach for converting binary data into a text format that can be easily communicated via text-based protocols such as HTTP. PHP provides functions that enable developers to encode and decode data using the Base64 technique, making it a useful tool for a variety of tasks such as data serialization, file attachments, and secure data transmission.
Base64 encoding is very beneficial in the context of PHP when working with binary data such as photos, audio files, and other multimedia information. It enables developers to convert such data into a format that can be readily incorporated within HTML, included in URLs, or transported between servers and clients without causing data corruption.
The following sections of this article will explore the Base64 encoding and decoding capabilities offered by PHP, along with practical examples demonstrating how to use these functions to handle various data manipulation scenarios.
Base64 Encoding in PHP: base64_encode
The base64_encode function in PHP is a core function that provides an easy way to execute Base64 encoding. This method accepts a string as input and returns its Base64-encoded version. When you need to convert binary data, like as images or files, into a textual representation that can be safely used in text-based formats like HTML, CSS, or XML, the base64_encode function is widely employed.
The syntax for using the base64_encode
function is straightforward:
$encodedData = base64_encode($data);
In this case, $data represents the binary data to be encoded. The function will return a Base64-encoded string, which you can then utilize in your application as needed.
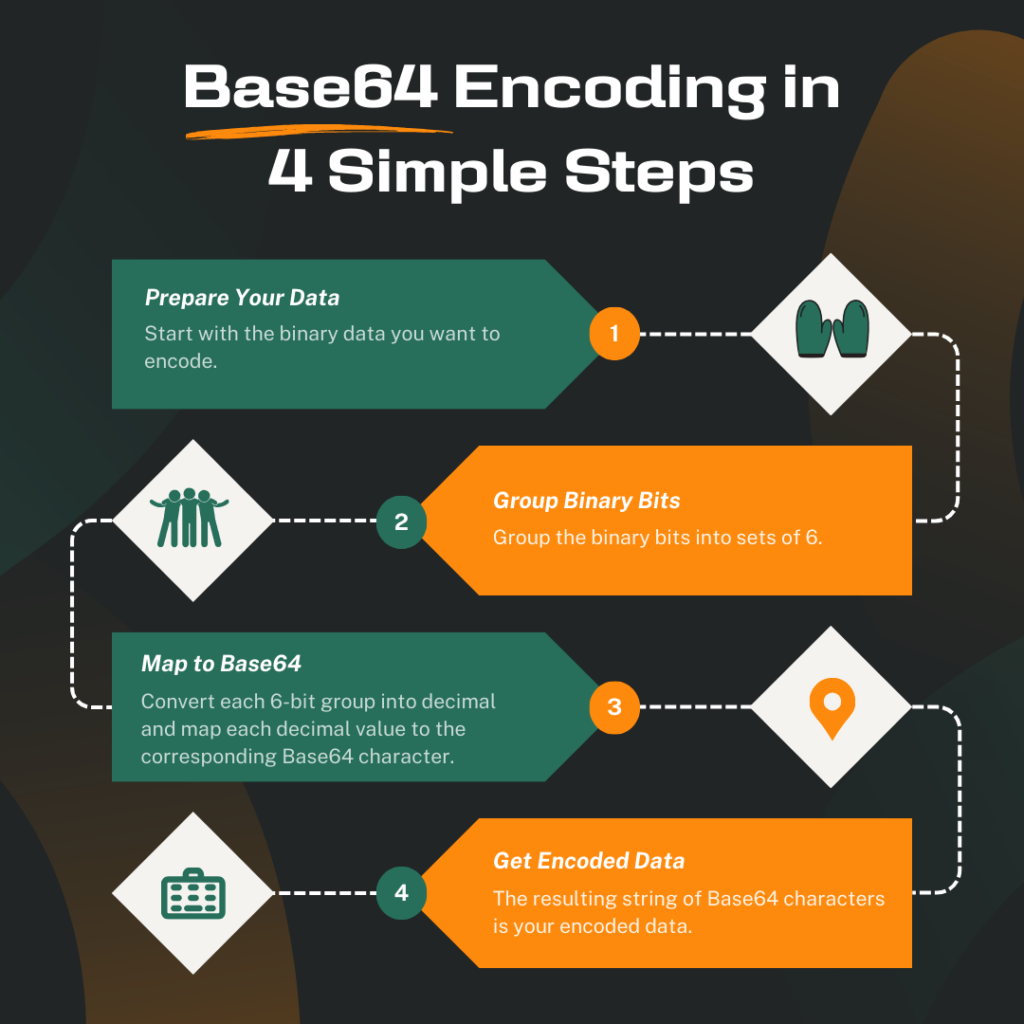
Example of Base64 Encoding in PHP
Assume you have an image file named “image.jpg” that you want to include in an HTML document. To convert an image file into a Base64-encoded string, use the base64_encode function:
$imagePath = 'path/to/image.jpg'; $imageData = file_get_contents($imagePath); $encodedImage = base64_encode($imageData);
Once the image data is encoded, you can include it in your HTML document as follows:
<img src="data:image/jpeg;base64,<?php echo $encodedImage; ?>" alt="Encoded Image">
In this example, the data
URI scheme is used to embed the Base64-encoded image directly within the HTML code.
In this lesson, you will learn more about using Base64 in an HTML environment.
Base64 Decoding in PHP: base64_decode
The base64_decode function in PHP is used to decode a Base64-encoded string back to its original binary contents. This method, which is the inverse of the base64_encode function, allows you to restore a Base64-encoded string to its original form, which is very handy when working with data that needs to be decoded for processing or storage.
The syntax for using the base64_decode
function is straightforward:
$decodedData = base64_decode($encodedData);
Here, $encodedData
represents the Base64-encoded string that you want to decode. The function will return the decoded binary data as a string.
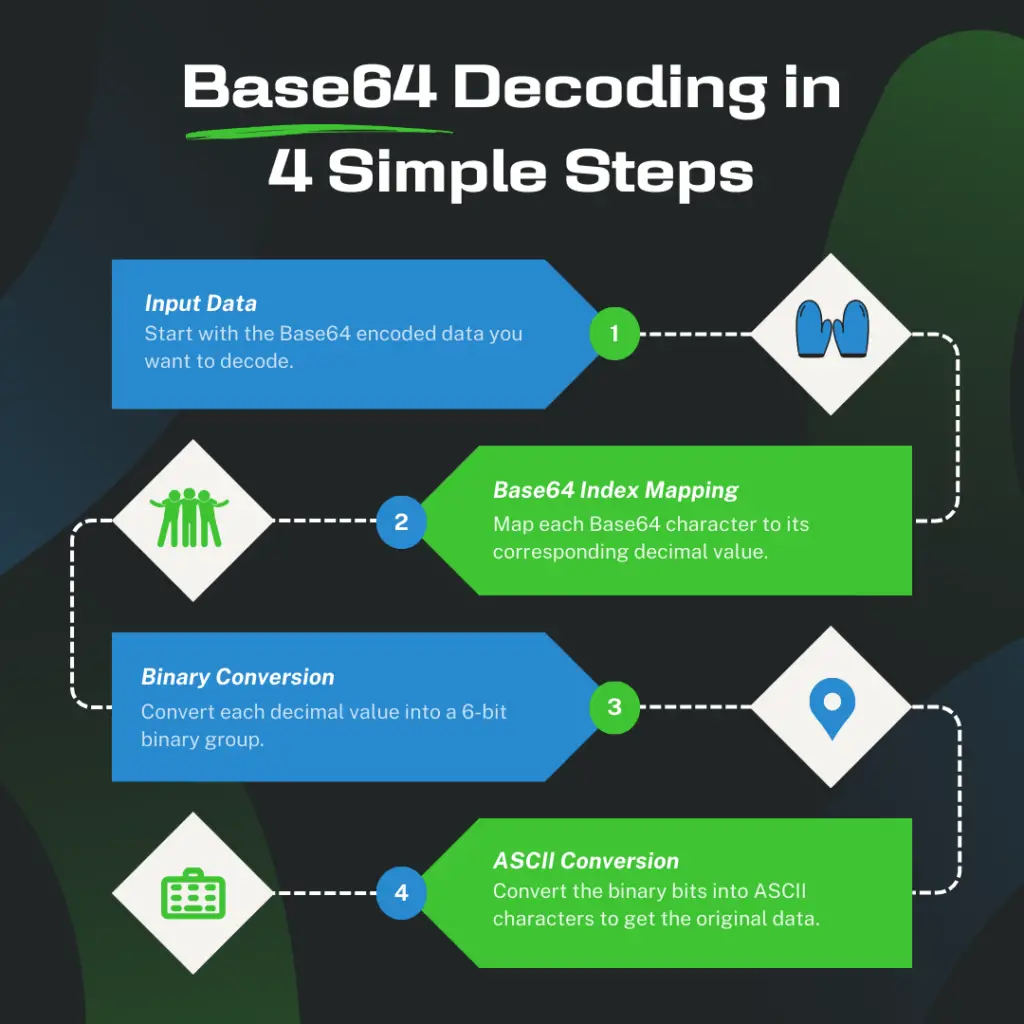
Example of Base64 Decoding in PHP
For example, if you receive a Base64-encoded string from a client and need to decode it in order to obtain the original data, use the base64_decode function as follows:
$encodedString = 'QjY0RW5jb2Rl'; $decodedData = base64_decode($encodedString);
In this example, a Base64 encoded string is assigned to the variable $encodedString
. The base64_decode
function is then used to decode the base64 encoded string and the result is stored in the variable $decodedData
. The base64_decode
function takes a Base64 encoded string as input and returns the decoded data .
After running this code, the value of $decodedData
will be the original data that was encoded into the Base64 string. In this case, the value of $decodedData
will be “B64Encode”.