Whether you’re an experienced C# developer or a beginner, this article will provide you with the information and practical examples you need to efficiently encode and decode data in C# using the Base64 algorithm. This article intends to equip you to work seamlessly with Base64-encoded data within the C# programming language, from comprehending the basics to hands-on demonstrations.
What is Base64?
Base64 is a binary-to-text encoding system that is often used to encode binary data into plain text format, such as photos, audio files, or other digital information.
Each group of three consecutive bytes from binary data is represented as four ASCII characters in the Base64 encoding scheme. These characters are drawn from a set of 64 (hence the name “Base64”), which contains 26 uppercase letters, 26 lowercase letters, 10 numerals, and the characters ‘+’ and ‘/’. Furthermore, if the length is not a multiple of three, the equals sign ‘=’ is utilized for padding at the end of the encoded data.
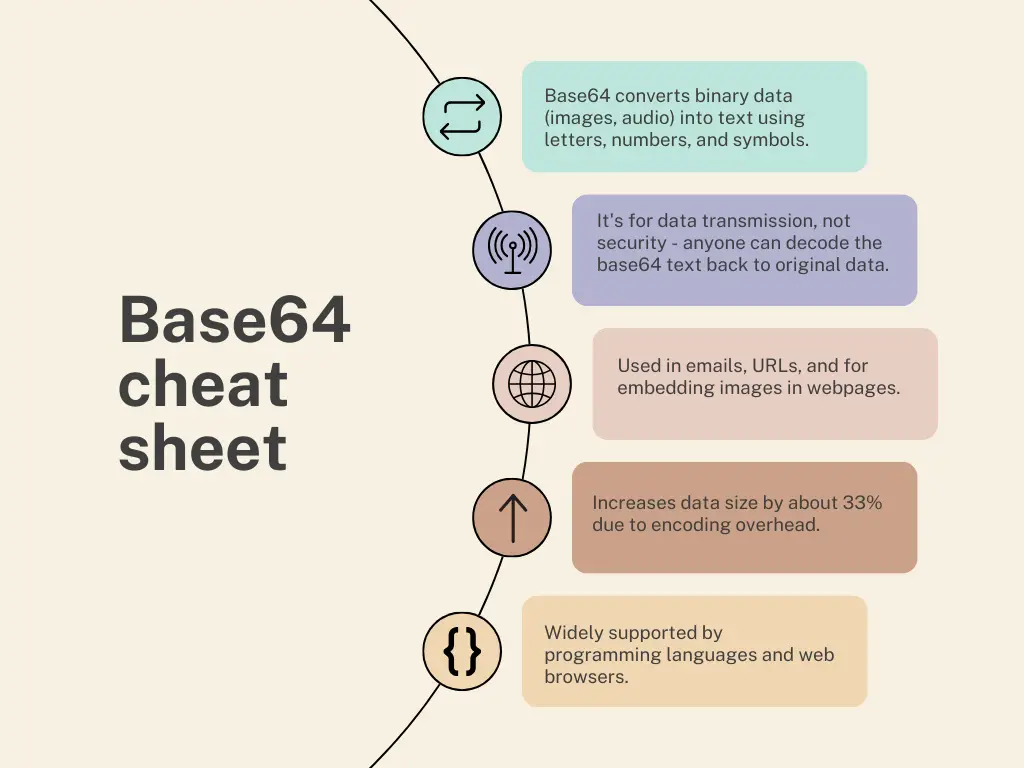
What is C#?
C# (or C Sharp) is a high-level, general-purpose programming language that supports several paradigms. It includes the programming disciplines of static typing, strong typing, lexically scoped, imperative, declarative, functional, generic, object-oriented (class-based), and component-oriented.
The C# programming language was created by Anders Hejlsberg from Microsoft in 2000 and was later approved as an international standard by Ecma (ECMA-334) in 2002 and ISO/IEC (ISO/IEC 23270) in 2003. It’s often used in developing software components suitable for deployment in distributed environments.
Here’s a quick summary:
- Modern & Object-Oriented: Designed for clear code structure and reusability, making development efficient.
- Powerful & Flexible: Handles complex tasks while offering control over system resources for performance.
- Popular Choice: Widely used for everything from game development to building robust software applications.
- Runs on .NET: Primarily designed for the .NET Framework, but also compatible with cross-platform .NET Core.
- Large Community & Resources: Benefits from a vast community of developers and extensive learning materials.
Does C# Support Base64?
Base64 encoding and decoding is a fundamental technique used in a variety of computer tasks, ranging from processing binary data in a human-readable format to data transmission across text-based protocols.
If you’re using C#, you’re in luck because the language has support for Base64 encoding and decoding. Through its standard libraries and methods, C# provides a simple and efficient approach to interact with Base64-encoded data. In the following sections, we’ll look at the native mechanisms that C# provides for dealing with Base64-encoded data, and how you can easily integrate Base64 operations into your programs.
Base64 Encoding in C#
In C#, you can use the Convert.ToBase64String method to encode a string into a Base64 string. This method is part of the System namespace and is available in the System.Runtime.dll assembly.
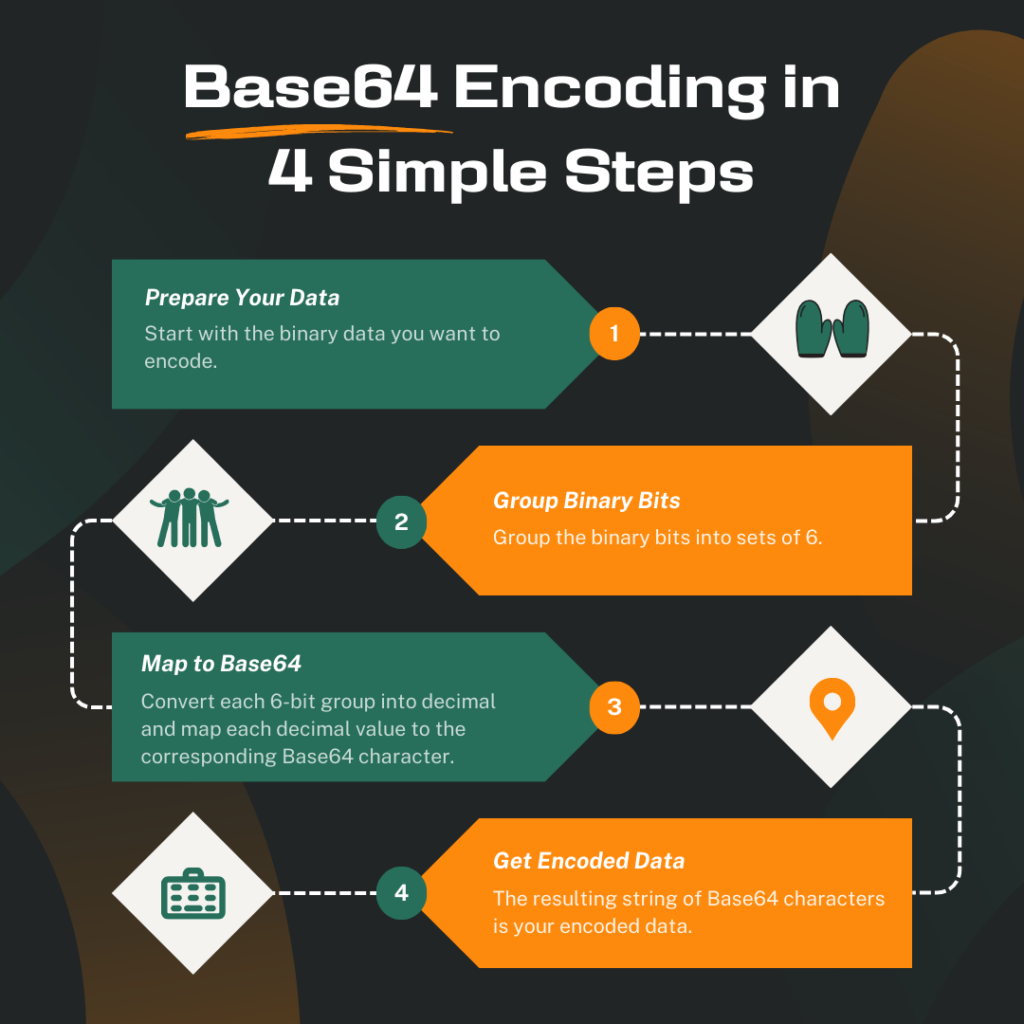
Example of Base64 Encoding in C#
Here’s an example of how you can use the Convert.ToBase64String
method to encode a string into a base64 string:
string originalString = "B64Encode"; byte[] originalBytes = Encoding.UTF8.GetBytes(originalString); string encodedString = Convert.ToBase64String(originalBytes);
In this example, we first convert the original string into an array of bytes using the Encoding.UTF8.GetBytes
method. Then, we pass this byte array to the Convert.ToBase64String
method to obtain the base64 encoded string.
Example of Converting File to Base64 in C#
Converting a file to a base64 string in C# is a straightforward process. First, you need to read the file into a byte array. This can be done using the File.ReadAllBytes
method, which is part of the System.IO
namespace. Once you have the byte array, you can pass it to the Convert.ToBase64String
method to obtain the base64 encoded string.
string filePath = @"C:\path\to\file"; byte[] fileBytes = File.ReadAllBytes(filePath); string base64String = Convert.ToBase64String(fileBytes);
In this example, we first specify the path of the file we want to convert. Then, we use the File.ReadAllBytes
method to read the file into a byte array. Finally, we pass this byte array to the Convert.ToBase64String
method to obtain the base64 encoded string.
This method may convert any form of file to a base64 string, including text, image, and audio files.
Base64 Decoding in C#
In C#, you can use the Convert.FromBase64String method to decode a base64 string into an array of bytes. This method is part of the System namespace and is available in the mscorlib.dll assembly.
To decode a base64 string into an array of bytes, you can pass the base64 string to the Convert.FromBase64String
method. Once you have the byte array, you may use the proper encoding, such as UTF-8, to convert it to a string.
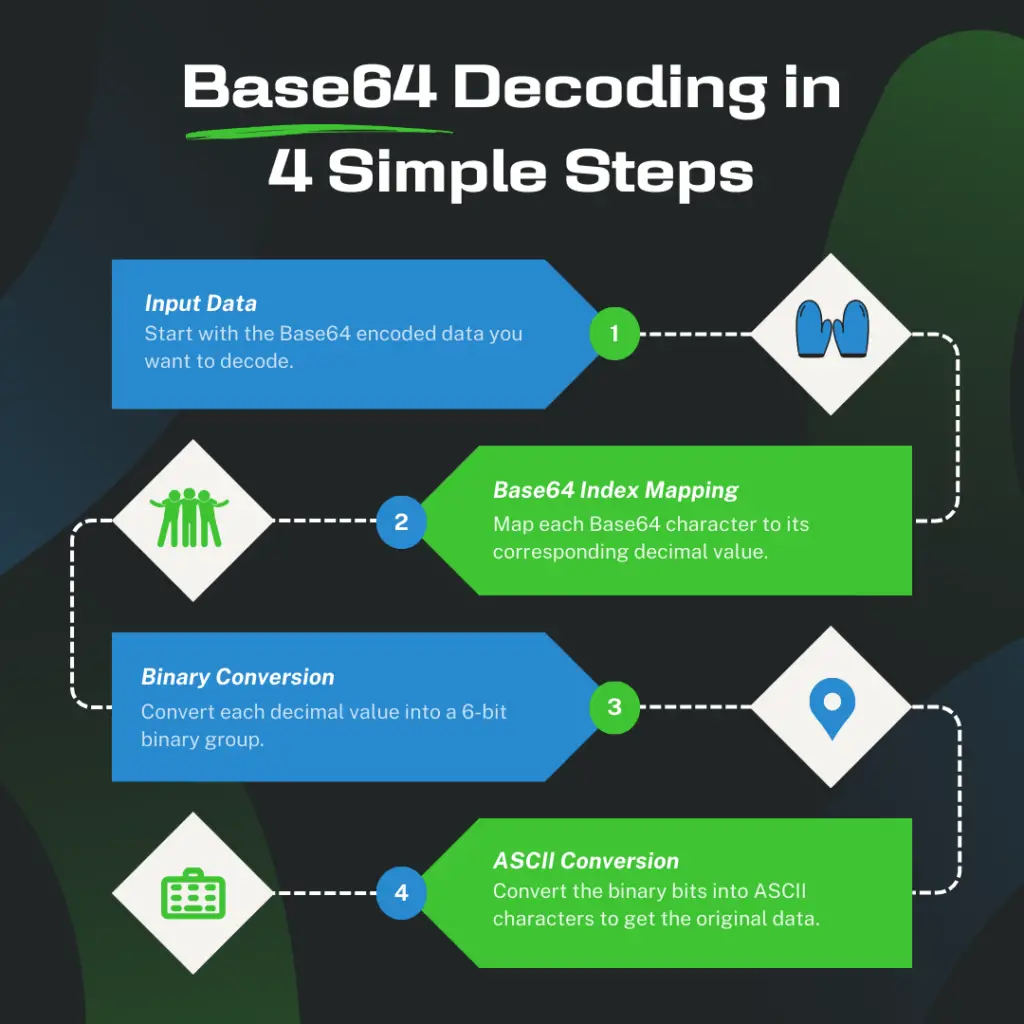
Example of Base64 Decoding in C#
In C#, decoding a Base64 string is a common task when working with binary data or encoded information. The language provides built-in functions to simplify this process. The Convert.FromBase64String()
method in C# is used to decode a Base64 string and convert it back to its original byte array representation. Here’s an example of how to use this function:
string base64String = "QjY0RW5jb2Rl"; byte[] decodedBytes = Convert.FromBase64String(base64String); string decodedString = Encoding.UTF8.GetString(decodedBytes);
In this example, we first specify the base64 string that we want to decode. Then, we use the Convert.FromBase64String
method to decode the base64 string into an array of bytes. Finally, we convert this byte array into a string using the Encoding.UTF8.GetString
method.
Decoding Base64 to File in C#
In C#, you can decode a Base64 string and write it to a file using the Convert.FromBase64String and File.WriteAllBytes methods.
Here’s an example:
string base64String = "QjY0RW5jb2Rl"; byte[] decodedBytes = Convert.FromBase64String(base64String); string filePath = @"C:\path\to\file"; File.WriteAllBytes(filePath, decodedBytes);
In this example, we first specify the base64 string that we want to decode. Then, we use the Convert.FromBase64String
method to decode the base64 string into an array of bytes. Finally, we specify the path of the file we want to write to and use the File.WriteAllBytes
method to write the decoded bytes to the file.