In this tutorial we’ll explore Base64URL encoding, its purpose, features and how it differs from regular Base64 encoding. Tailored for online and URL use, Base64URL overcomes the limitations of its regular counterpart. We’ll delve into the details of this encoding scheme, including its character set and padding technique. By the end of this article, you will have a thorough understanding of Base64URL encoding and how to implement it in your programming language.
What is Base64URL?
Base64URL is a specific form of the well-known Base64 encoding system in the world of data encoding. Its sole goal is to accommodate the requirements and constraints given by web environments and URLs.
Base64URL is a mechanism for encoding binary data into a text-based format suitable for web transmission. This encoding not only ensures data integrity during transmission, but it also tackles compatibility concerns that may arise when non-ASCII characters are used in URLs.
Base64URL uses a slightly different character set and padding method than regular Base64 encoding. Because of these changes, it is excellent for situations where regular Base64 encoded data might cause problems, such as when data is included in URLs, query parameters, or path segments.
In this article, we’ll look at the complexities of Base64 URL encoding, its underlying character set, padding schemes, and so on.
What’s the Difference Between Base64URL and Base64? Base64URL vs Base64
While both Base64 and Base64URL have the same basic purpose of encoding binary data into a text-based format, they cater to different settings and usage scenarios, which results in numerous important variances in their implementation and characteristics.
- Character Set: Base64 employs a character set that includes special characters such as “+”, “/”, and “=”, which might cause problems in URL situations due to their unique meanings. Base64URL, on the other hand, uses a URL-safe character set by replacing “+” with “-“, “/” with “_”, and omitting padding with “=”.
- Padding: Padding characters (“=”) are used by Base64 to ensure that the encoded data length is a multiple of four. Base64URL, on the other hand, removes all padding, resulting in a more compact representation and avoiding potential URL issues.
- URL Compatibility: When using Base64-encoded data in URLs, the special characters can be misread or modified by web servers and browsers. Base64URL, which was created with URLs in mind, overcomes these compatibility difficulties by using URL-safe characters.
- Encoding Purpose: Base64 is utilized more generally, embracing data encoding for a variety of purposes. Base64URL, on the other hand, is specifically designed for web applications and instances where data must be contained in URLs or securely communicated over the web.
Base64 and Base64URL differ primarily in their character sets, padding mechanisms, and compatibility with web environments. Understanding these distinctions allows developers to select the best encoding method for their individual use case, ensuring flawless data representation and transmission in their applications.
Below are the characters in which Base64 and Base64URL differ:
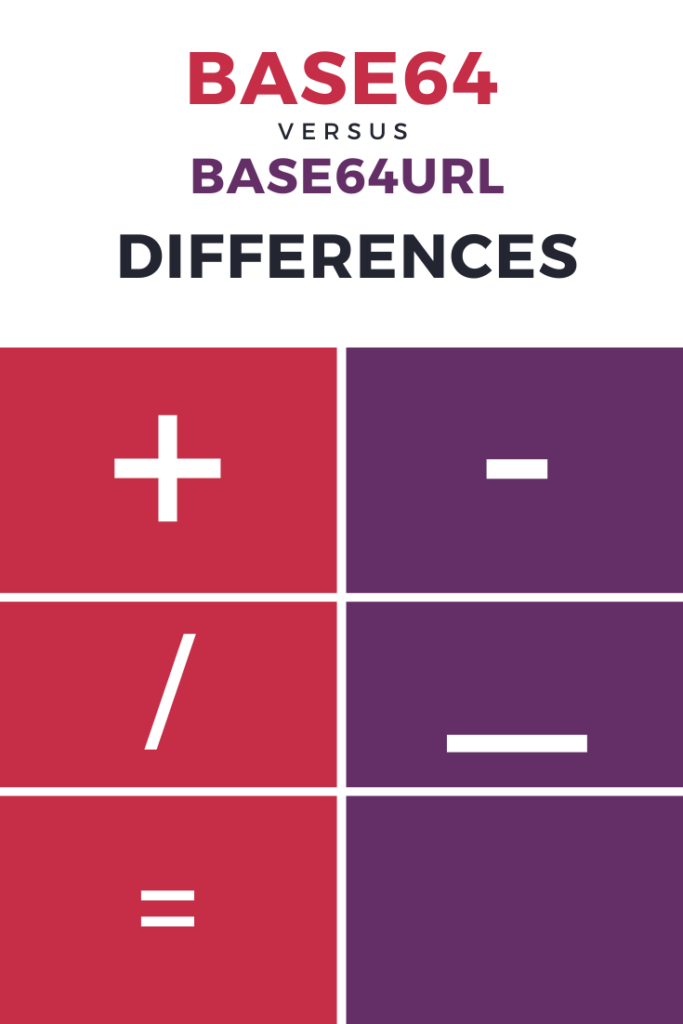
Now in table format:
Base64 Character | Base64URL Equivalent |
---|---|
+ | – |
/ | _ |
= (Padding) | (Padding omitted) |
Base64URL Character Set
Following that, we’ll walk over the whole Base64URL character set, providing you with an understanding of the characters used to encode binary data in a URL-safe format. This table shows how Base64URL provides secure and efficient data encoding within online contexts and URLs by illustrating the link between decimal numbers and their equivalent characters.
Decimal | Character |
---|---|
0 | A |
1 | B |
2 | C |
3 | D |
4 | E |
5 | F |
6 | G |
7 | H |
8 | I |
9 | J |
10 | K |
11 | L |
12 | M |
13 | N |
14 | O |
15 | P |
16 | Q |
17 | R |
18 | S |
19 | T |
20 | U |
21 | V |
22 | W |
23 | X |
24 | Y |
25 | Z |
26 | a |
27 | b |
28 | c |
29 | d |
30 | e |
31 | f |
32 | g |
33 | h |
34 | i |
35 | j |
36 | k |
37 | l |
38 | m |
39 | n |
40 | o |
41 | p |
42 | q |
43 | r |
44 | s |
45 | t |
46 | u |
47 | v |
48 | w |
49 | x |
50 | y |
51 | z |
52 | 0 |
53 | 1 |
54 | 2 |
55 | 3 |
56 | 4 |
57 | 5 |
58 | 6 |
59 | 7 |
60 | 8 |
61 | 9 |
62 | – |
63 | _ |
Padding in Base64URL
Unlike traditional Base64 encoding, Base64URL employs specific padding and trimming strategies.
In standard Base64 encoding, padding is denoted by the “=” character, ensuring that the encoded data length is a multiple of four. In Base64URL, padding is omitted altogether. The absence of padding not only reduces the encoded data’s length but also simplifies its integration within URLs and other web-related structures.
Base64URL in Programming Languages
While most programming languages do not natively offer Base64URL encoding and decoding, it is possible with small adjustments. Let’s look at how this process works and give an example in a popular programming language.
In languages without built-in Base64URL support, you have to replace the characters “+” with “-“, “/” with “_”, and remove “=” padding characters to create a URL-safe character set.
import base64 def base64url_encode(data): # Encode the data using base64 encoded = base64.b64encode(data) # Replace characters that are not URL safe with URL safe characters url_safe_encoded = encoded.replace(b'+', b'-').replace(b'/', b'_').rstrip(b'=') return url_safe_encoded def base64url_decode(encoded): # Add padding if necessary padding = b'=' * (4 - (len(encoded) % 4)) # Replace URL safe characters with their original characters url_safe_encoded = encoded.replace(b'-', b'+').replace(b'_', b'/') + padding # Decode the data using base64 decoded = base64.b64decode(url_safe_encoded) return decoded # Example usage data_to_encode = b"Data to Encode." encoded_data = base64url_encode(data_to_encode) decoded_data = base64url_decode(encoded_data) print("Original Data:", data_to_encode) print("Encoded Data:", encoded_data) print("Decoded Data:", decoded_data)