This post will show you how to use Base64 to effectively encode and decode data. We’ll look at practical examples like string encoding and file conversion. By the conclusion, you’ll be able to use Base64 with confidence in your web development projects.
What is Base64?
Base64 is a binary-to-text encoding system that converts binary data into an ASCII string format that can be printed. It’s frequently used to represent data in a way that’s safe for transmission across text-based protocols. This encoding allows binary data, such as images or files, to be communicated as text characters in an efficient manner. Understanding Base64 is necessary for several data processing tasks and web development apps in JavaScript.
Common Applications of Base64 Encoding in Web Development
Base64 encoding is useful in a variety of web development scenarios, including:
- Images in CSS: Embedding images as Base64-encoded strings directly into CSS files, eliminating the need for separate image files.
- Data URLs: Data URLs use Base64 encoding to integrate images or resources directly within HTML.
- Cookies: Binary data such as pictures or files are stored as Base64-encoded strings within cookies.
- File Uploads: Uploading files from the client to the server via AJAX requests or form submissions.
- API Communication: API communication refers to the transmission of binary data as part of API calls, particularly when dealing with media or file uploads.
Developers can improve the overall performance and efficiency of web applications by using Base64 encoding to optimize data handling and reduce the number of external file requests.
What is JavaScript?
If you’ve made it this far, you’re probably aware that JavaScript, or JS for short, is a popular computer language known for its use in web development. It provides dynamic and interactive functionality on websites. While we won’t go into detail, JavaScript’s interoperability with Base64 encoding and decoding makes it an important player in data processing and transfer.
Does JavaScript Support Base64?
Absolutely. JavaScript includes methods for Base64 encoding and decoding. These technologies provide a straightforward way to manipulate data by transforming binary information into a plain text format that is easily shareable and transportable. With these utilities, you can easily include Base64 encoding and decoding into your JavaScript projects, easing data conversion and transmission operations.
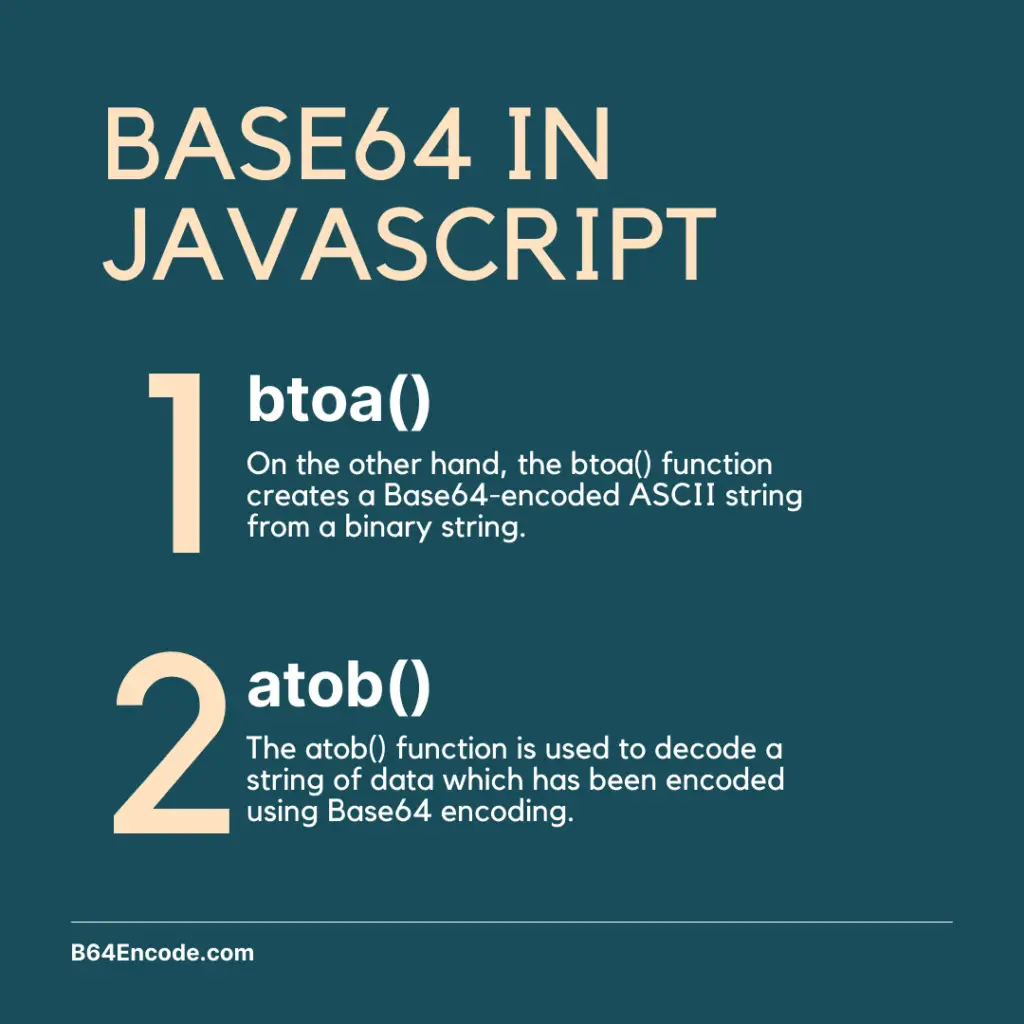
Base64 Encoding in JavaScript using btoa() Function
JavaScript includes a method called btoa(), which stands for “binary to ASCII“. This method makes it simple to encode binary data into Base64 format, such as strings or binary files. It’s a simple procedure in which the btoa() method takes binary data as input and returns a Base64-encoded text.
In the following part, we’ll look at how to use the btoa() method to encode various forms of data and look at practical examples that show its adaptability in web development.
Example of Base64 Encoding in JavaScript
Let’s look at a basic example to see how the btoa() method in JavaScript works for Base64 encoding.
Assume you have a string that says, “Hello, World!” to encode this string with btoa(), provide it to the function as an argument:
// Declare a variable to hold the original string const originalString = Hello, World!; // Use the btoa() function to encode the original string in Base64 const encodedString = btoa(originalString); // Output: "SGVsbG8sIFdvcmxkIQ" console.log(encodedString);
The resulting encodedString will contain the Base64-encoded version of the original string:
SGVsbG8sIFdvcmxkIQ==
This encoded string can now be easily shared or transmitted without concern about incompatibility.
Example of Converting Files/Images to Base64 in JavaScript
Converting files to Base64 encoding is a typical process in web development, and it is frequently used for scenarios such as AJAX file uploads or embedding images straight into HTML. Let’s look at a simple JavaScript sample to see how this process works.
Assume you have a “image.png” picture file that you want to convert to Base64 format. Here’s how you do it with the FileReader API and the btoa() function:
// Select the input element where the user uploads the file const input = document.querySelector('input[type="file"]'); // Listen for the 'change' event when a file is selected input.addEventListener('change', (event) => { const file = event.target.files[0]; // Use FileReader to read the file as a Data URL const reader = new FileReader(); reader.onload = (readerEvent) => { const dataURL = readerEvent.target.result; // Remove the "data:image/png;base64," prefix const base64Data = dataURL.split(',')[1]; // Encode the Base64 data const encodedData = btoa(base64Data); // Now 'encodedData' contains the Base64-encoded file data }; // Read the file as a Data URL reader.readAsDataURL(file); });
Here is what happens in the code above:
- The
input
variable is assigned the result of callingdocument.querySelector()
with the argument'input[type="file"]'
. This selects the<input type="file">
element on the page. - When the user picks a file, an event listener is attached to the input element to listen for the ‘change’ event.
- When the
'change'
event is triggered, the first file in theevent.target.files
array is assigned to thefile
variable. - The reader variable is assigned a new FileReader object that is created.
- An event listener is added to the
reader
object to listen for the'load'
event, which is triggered when the file has been read as a Data URL. - When the
'load'
event is triggered, the result of reading the file as a Data URL is assigned to thedataURL
variable. - The
"data:image/png;base64,"
prefix is removed from thedataURL
by calling.split(',')[1]
, and the result is assigned to thebase64Data
variable. - The
base64Data
variable is then passed as an argument to thebtoa()
function, which encodes it in Base64 and returns the result. This result is assigned to theencodedData
variable. - Finally, the
reader.readAsDataURL()
method is called with thefile
variable as an argument, which starts reading the file as a Data URL.
Base64 Decoding in JavaScript using atob() Function
In addition to encoding, JavaScript includes an atob() function for decoding Base64-encoded strings. This function, which stands for “ASCII to binary,” is the inverse of the btoa() function. It accepts as input a Base64-encoded string and returns the original binary data.
When you need to decode data that was previously encoded using the btoa() function, the atob() function comes in handy. This method is very handy when getting data in Base64 format and needs to convert it back to its original form for further processing.
Example of Base64 Decoding in JavaScript
Let’s use a simple example to demonstrate the process of Base64 decoding with the atob() function.
Assume you have the following Base64-encoded string: “SGVsbG8sIFdvcmxkIQ==“, which corresponds to the original string “Hello, World!” You may use the atob() method to decode this Base64-encoded text and obtain the original data:
// Declare a variable to hold the Base64-encoded string const encodedString = "SGVsbG8sIFdvcmxkIQ=="; // Use the atob() function to decode the Base64-encoded string const decodedString = atob(encodedString); // Log the decoded string to the console console.log(decodedString); // Output: Hello, World!
Decoding Base64 to File/Image in JavaScript
Decoding Base64-encoded data back to its original file or picture format is a typical operation in web development, and it is frequently employed when dealing with file uploads or retrieving embedded images. Let’s look at an example that shows how to use JavaScript to decode a Base64-encoded image and display it on a webpage.
Assume you have a Base64-encoded image string and wish to display it on an HTML element with the ID “image-container“:
// This is our Base64 Image const encodedImage = "R0lGODlhAQABAIAAAP///wAAACH5BAEAAAAALAAAAAABAAEAAAICRAEAOw=="; // Create a new image element const img = document.createElement("img"); // Set the source of the image to the decoded Base64 data img.src = "data:image/png;base64," + encodedImage; // Append the image to the designated container const imageContainer = document.getElementById("image-container"); imageContainer.appendChild(img);
The following step-by-step guide explains the above code:
- A value representing a Base64-encoded image string is allocated to the encodedImage variable.
- A new
<img>
element is created by callingdocument.createElement("img")
and the result is assigned to theimg
variable. - The
src
attribute of theimg
element is set to a data URL that represents the decoded Base64 image data. This is done by concatenating the string"data:image/png;base64,"
with the value of theencodedImage
variable. - The
imageContainer
variable is assigned the result of callingdocument.getElementById("image-container")
, which selects an element on the page with anid
attribute of"image-container"
. - The
img
element is appended to theimageContainer
element as a child by callingimageContainer.appendChild(img)
.
How to Download Base64 File in JavaScript Example
If you need to download the Base64 content, don’t worry, you can easily do that in JavaScript. Assume you have a Base64-encoded file and want customers to be able to download it when they click the “download-button” button:
const encodedFile = "YjY0ZW5jb2RlLmNvbQ=="; // Select the download button element const downloadButton = document.getElementById("download-button"); // Add a click event listener to the button downloadButton.addEventListener("click", () => { // Create a new Blob from the decoded Base64 data const blob = new Blob([Uint8Array.from(atob(encodedFile), c => c.charCodeAt(0))]); // Create a download URL for the Blob const downloadUrl = URL.createObjectURL(blob); // Create a temporary link element const link = document.createElement("a"); link.href = downloadUrl; link.download = "downloaded-file.txt"; // Set the desired file name link.click(); // Clean up the URL and the temporary link URL.revokeObjectURL(downloadUrl); });
Detailed explanation of the above code:
- The encodedFile variable receives a value representing a Base64-encoded file.
- The
downloadButton
variable is assigned the result of callingdocument.getElementById("download-button")
, which selects an element on the page with anid
attribute of"download-button"
. - When the user clicks the button, an event listener is attached to the downloadButton element to listen for the ‘click’ event.
- When the
'click'
event is triggered, a newBlob
object is created from the decoded Base64 data by callingnew Blob([Uint8Array.from(atob(encodedFile), c => c.charCodeAt(0))])
. ThisBlob
object represents the contents of the file to be downloaded. - A download URL for the
Blob
object is created by callingURL.createObjectURL(blob)
, and the result is assigned to thedownloadUrl
variable. - A temporary
<a>
element is created by callingdocument.createElement("a")
, and itshref
attribute is set to the value of thedownloadUrl
variable. - The click() method of the <a> element is invoked, which starts the file download.
- The download URL is revoked by calling
URL.revokeObjectURL(downloadUrl)
. - The
download
attribute of the<a>
element is set to"downloaded-file.txt"
, which specifies the desired file name for the downloaded file.