Base64 encoding plays a crucial role in web development, enabling the safe and efficient transmission of binary data within text-based environments. This article delves into the mechanics of Base64 within the context of Angular applications. We will also show you how to use two packages: ng2-pdf-viewer
and ng2-pdfjs-viewer
.
What is Base64?
Base64 encoding is a ubiquitous technique employed in computer systems to effectively represent binary data, such as images or files, within the confines of text-based environments. This ASCII-based approach facilitates the secure transmission of binary information across communication channels that are restricted to handling text-only formats.
The core principle of Base64 encoding lies in its ability to convert binary data into a sequence of printable ASCII characters. This conversion process involves splitting the binary data into groups of three bytes (24 bits). Each of these 24-bit groups is then mapped to a specific combination of four ASCII characters from a defined set of 64 unique characters. This mapping ensures that the encoded data remains compatible with text-based protocols and can be safely transmitted without corruption.
Therefore, Base64 encoding acts as a crucial bridge between the binary world of data and the text-based realm of communication. It empowers developers to seamlessly exchange binary information within environments that are inherently limited to handling text-only content.
What is Angular?
Google’s Angular is a popular JavaScript framework for creating dynamic web applications. It offers capabilities such as data binding, dependency injection, and modular components in a structured framework for developing single-page applications. Angular‘s broad ecosystem and tools make it easier to create complex and engaging user experiences.
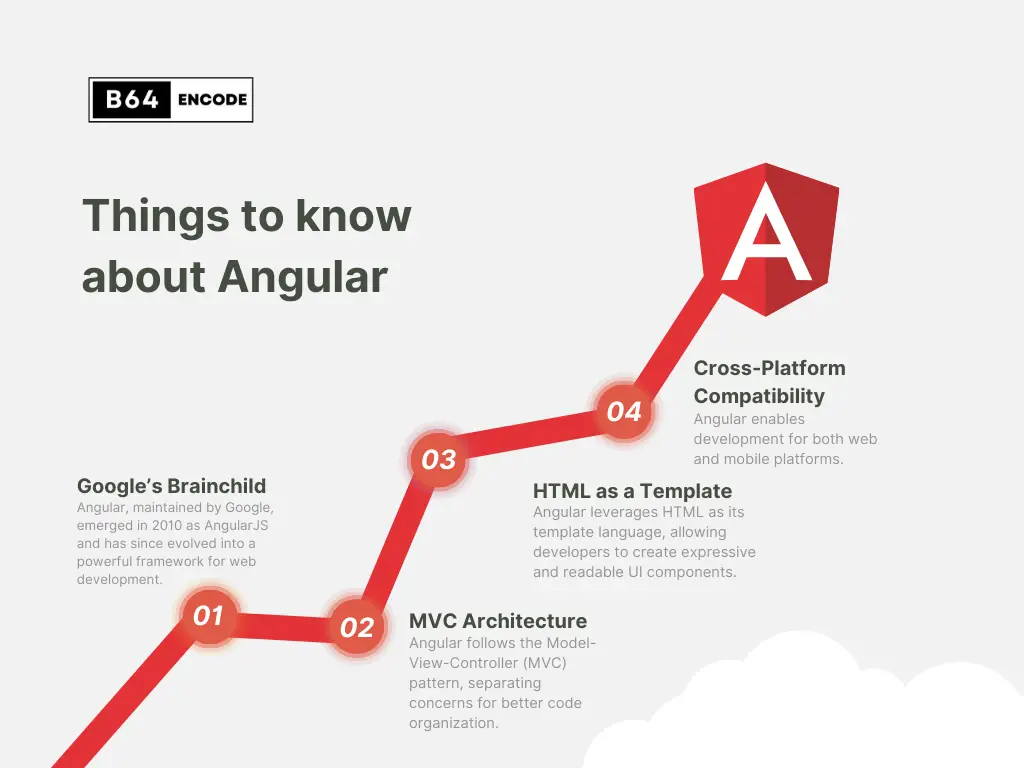
In the intricate landscape of modern web applications, efficient management of dependencies becomes a critical factor. Angular rises to this challenge with its powerful dependency injection mechanism, enabling developers to explicitly declare their application’s dependencies. This proactive approach fosters a codebase characterized by enhanced modularity, maintainability, and scalability. By meticulously injecting services precisely where they are required, Angular promotes a clean separation of concerns, ensuring that each component operates independently while contributing effectively to the overall application’s efficiency.
Beyond its dependency management capabilities, Angular’s component-based architecture lays the foundation for the development of reusable and self-contained UI elements. This design philosophy, which encapsulates logic, templates, and styling within each component, reinforces modularity and reusability throughout the application. From navigation bars and forms to data visualization widgets, components empower developers to effortlessly construct sophisticated user interfaces, streamlining the development process and ensuring consistency across the application.
Furthermore, Angular’s structured framework is meticulously tailored for Single-Page Applications (SPAs). SPAs deliver a seamless, app-like experience by loading a single HTML page and dynamically updating content in response to user interactions. Angular’s dedicated routing module streamlines navigation within the application, granting developers the ability to define routes, implement lazy loading of modules, and manage deep linking with ease. This inherent focus on SPAs positions Angular as a powerful and versatile development tool that caters to the specific requirements of modern web applications.
Base64 Encoding and Decoding in Angular
Base64 encoding and decoding play a crucial role in seamlessly transforming binary data into a text-based format suitable for various communication and storage scenarios. Within the realm of Angular development, these operations can be readily achieved using the framework’s built-in JavaScript functions.
In Angular, you can perform Base64 encoding using the btoa()
function, which stands for “binary to ASCII”. This function takes binary data as input and returns a Base64-encoded string. For example, to encode a string, you can call the btoa()
function with the string as an argument:
const originalString = 'B64Encode.com'; const encodedString = btoa(originalString); console.log(encodedString);
To decode a Base64-encoded string back to its original binary form, you can use the atob()
function, which stands for “ASCII to binary.” This function takes a Base64-encoded string as input and returns the original binary data. Here’s an example:
const encodedString = "QjY0RW5jb2RlLmNvbQ=="; const decodedString = atob(encodedString); console.log(decodedString);
Example: PDF to Base64 in Angular
In this example, we create an Angular component named PdfToBase64Component
. The component includes an input element for selecting a PDF file and a button to trigger the conversion process. When a file is selected, the handleFileInput()
method is invoked, which reads the file and uses a FileReader to convert it to a Base64-encoded string. The Base64-encoded string is then displayed in a textarea element.
import { Component } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-pdf-to-base64', template: ` <input type="file" (change)="handleFileInput($event)" accept=".pdf" /> <button (click)="convertToBase64()">Convert to Base64</button> <div *ngIf="base64String"> <h3>Base64-encoded PDF content:</h3> <textarea rows="10">{{ base64String }}</textarea> </div> `, }) export class PdfToBase64Component { base64String: string | null = null; constructor(private http: HttpClient) {} handleFileInput(event: any): void { const file = event.target.files[0]; if (file) { this.convertFileToBase64(file); } } convertFileToBase64(file: File): void { const reader = new FileReader(); reader.onload = (event: any) => { this.base64String = event.target.result.split(',')[1]; }; reader.readAsDataURL(file); } convertToBase64(): void { // Perform additional operations if needed } }
Example: Base64 to PDF in Angular
The user inputs the Base64-encoded PDF information into a textarea in this component. When the “Convert to PDF” button is clicked, the convertToPdf()
method is called. This method decodes the Base64 content using the atob()
function and creates a Uint8Array
from the decoded binary data.
import { Component } from '@angular/core'; @Component({ selector: 'app-base64-to-pdf', template: ` <textarea placeholder="Enter Base64-encoded PDF content" [(ngModel)]="base64Content" rows="10" ></textarea> <button (click)="convertToPdf()">Convert to PDF</button> <div *ngIf="pdfBlobUrl"> <h3>Converted PDF:</h3> <object [data]="pdfBlobUrl" type="application/pdf" width="100%" height="500px"> Your browser does not support PDF embedding. </object> </div> `, }) export class Base64ToPdfComponent { base64Content: string = ''; pdfBlobUrl: string | null = null; convertToPdf(): void { if (this.base64Content) { const binaryData = atob(this.base64Content); const byteArray = new Uint8Array(binaryData.length); for (let i = 0; i < binaryData.length; i++) { byteArray[i] = binaryData.charCodeAt(i); } const blob = new Blob([byteArray], { type: 'application/pdf' }); this.pdfBlobUrl = URL.createObjectURL(blob); } } }
Angular PDF Base64 Viewer: ng2-pdf-viewer
Here’s an example of an Angular PDF viewer that uses ng2-pdf-viewer to display a PDF file from a Base64-encoded string:
npm install ng2-pdf-viewer
Create a new component named PdfViewerComponent
:
import { Component, Input } from '@angular/core'; @Component({ selector: 'app-pdf-viewer', template: ` <div *ngIf="pdfSrc"> <pdf-viewer [src]="pdfSrc" [render-text]="true" style="display: block;"></pdf-viewer> </div> `, }) export class PdfViewerComponent { @Input() pdfSrc: string | undefined; }
Use the PdfViewerComponent
in your main application component (AppComponent
):
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Angular PDF Viewer Base64 Example</h1> <app-pdf-viewer [pdfSrc]="base64Pdf"></app-pdf-viewer> `, }) export class AppComponent { base64Pdf = '...'; // Replace with your Base64-encoded PDF content }
In the above example, the PdfViewerComponent
takes an input property pdfSrc
, which should be the Base64-encoded PDF content. The ng2-pdf-viewer
package handles rendering the PDF using the provided Base64 data.
Remember to include the PdfViewerModule
in your AppModule
:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { PdfViewerModule } from 'ng2-pdf-viewer'; // Import PdfViewerModule import { PdfViewerComponent } from './pdf-viewer.component'; // Import PdfViewerComponent import { AppComponent } from './app.component'; @NgModule({ imports: [ BrowserModule, PdfViewerModule, // Add PdfViewerModule ], declarations: [ AppComponent, PdfViewerComponent, // Add PdfViewerComponent ], bootstrap: [AppComponent] }) export class AppModule { }
Replace '...'
in the base64Pdf
property of the AppComponent
with your actual Base64-encoded PDF content.
Angular PDF Base64 Viewer: ng2-pdfjs-viewer
Here’s how you can create an Angular PDF Base64 Viewer using the ng2-pdfjs-viewer
package.
First, install the ng2-pdfjs-viewer
package by running the following command:
npm install ng2-pdfjs-viewer
Create a new component named PdfBase64ViewerComponent
:
import { Component, Input } from '@angular/core'; @Component({ selector: 'app-pdf-base64-viewer', template: ` <div *ngIf="pdfSrc"> <ng2-pdfjs-viewer [pdfSrc]="pdfSrc" [show-all]="true"></ng2-pdfjs-viewer> </div> `, }) export class PdfBase64ViewerComponent { @Input() pdfSrc: string | undefined; }
Use the PdfBase64ViewerComponent
in your main application component (AppComponent
):
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Angular PDF Base64 Viewer: ng2-pdfjs-viewer Example</h1> <app-pdf-base64-viewer [pdfSrc]="base64Pdf"></app-pdf-base64-viewer> `, }) export class AppComponent { base64Pdf = '...'; // Replace with your Base64-encoded PDF content }
Include the PdfJsViewerModule
in your AppModule
:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { PdfJsViewerModule } from 'ng2-pdfjs-viewer'; // Import PdfJsViewerModule import { PdfBase64ViewerComponent } from './pdf-base64-viewer.component'; // Import PdfBase64ViewerComponent import { AppComponent } from './app.component'; @NgModule({ imports: [ BrowserModule, PdfJsViewerModule, // Add PdfJsViewerModule ], declarations: [ AppComponent, PdfBase64ViewerComponent, // Add PdfBase64ViewerComponent ], bootstrap: [AppComponent] }) export class AppModule { }
Replace '...'
in the base64Pdf
property of the AppComponent
with your actual Base64-encoded PDF content.
How to Open Base64 PDF in New Tab
This code snippet demonstrates how to open a Base64-decoded PDF in a new tab or window.
openPdfInNewTab(): void { if (this.base64Pdf) { const byteCharacters = atob(this.base64Pdf); const byteNumbers = new Array(byteCharacters.length); for (let i = 0; i < byteCharacters.length; i++) { byteNumbers[i] = byteCharacters.charCodeAt(i); } const byteArray = new Uint8Array(byteNumbers); const blob = new Blob([byteArray], { type: 'application/pdf' }); const blobUrl = URL.createObjectURL(blob); window.open(blobUrl, '_blank'); // This opens the new tab } }
Window.open(blobUrl, '_blank')
creates a new tab with the Blob URL representing the Base64-encoded PDF content. The '_blank'
parameter indicates to the window.open()
function to open the provided URL in a new tab.