Mastering Base64 encoding and decoding is a crucial skill for any Dart and Flutter developer, as it enables seamless data manipulation and transfer in various applications.
By learning to transform binary data into a more comprehensible form, you can enhance your ability to work with images, files, and other data types, ultimately improving the performance and functionality of your Dart and Flutter projects.
Discover the power of Base64 encoding and decoding in the Dart programming language and Flutter. Learn to easily transform and interpret binary data into concrete and easy-to-understand form.
What is Base64?
Base64 is a method for converting binary data into a textual representation that is universally supported in digital communication. This encoding scheme turns binary data into a string of ASCII characters, allowing it to be transmitted across text-only channels. Base64 provides data sharing without the possibility of special characters causing problems, making it a critical tool for data exchange across multiple technologies.
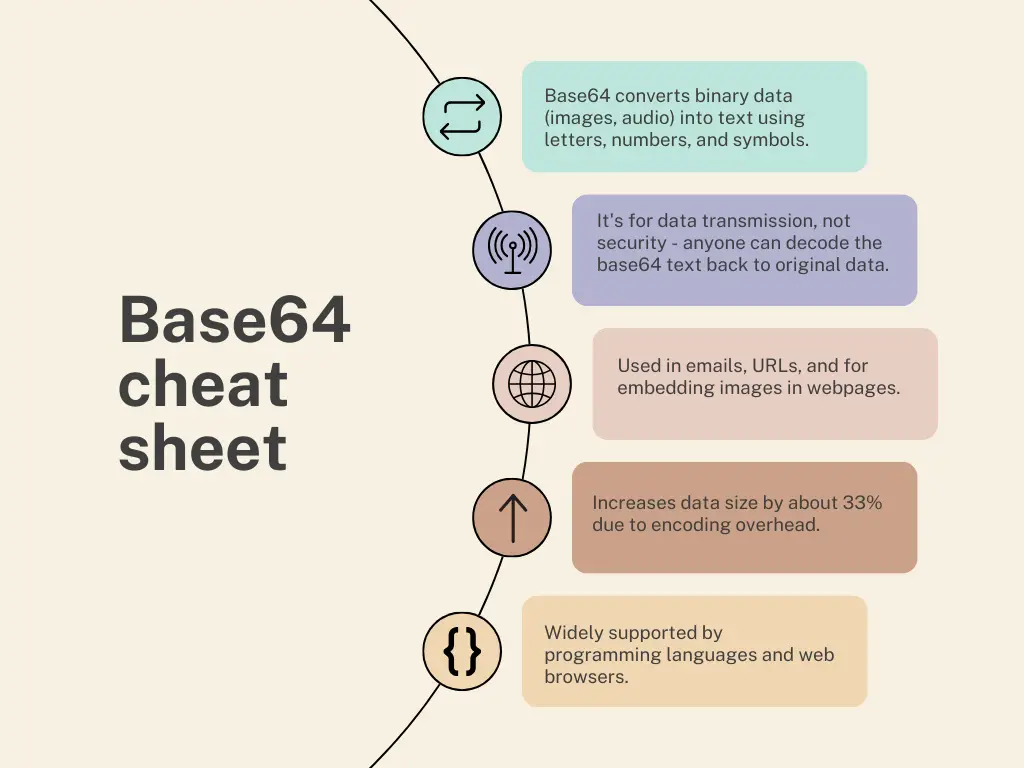
What is Dart?
Dart is a programming language created by Google that is used to create online and mobile applications. It is most often connected with the Flutter framework, which is used to develop cross-platform mobile apps. Dart prioritizes efficiency and performance, with features like as a strong type system, asynchronous programming, and a Just-In-Time (JIT) compiler that enables rapid development.
Here’s a quick breakdown:
- Versatile: Develops web, mobile, desktop, and even server-side applications.
- Easy to Learn: Uses a clean and familiar syntax, making it approachable for beginners.
- Fast & Efficient: Creates high-performing apps with features like hot reload for quick updates.
- Object-Oriented: Organizes code effectively using objects and classes.
- Powers Flutter: The Flutter framework is the foundation for building beautiful mobile apps.
What is Flutter?
Flutter is a Google-created open-source UI (User Interface) software development platform. It is designed to help developers build natively compiled applications for mobile, web, and desktop from a single codebase. Flutter is particularly known for its fast development process, expressive and flexible user interfaces, and native performance on various platforms.
Flutter’s core programming language is Dart.
A brief overview:
- Build Once, Run Anywhere: Create beautiful apps for mobile (Android, iOS), web, desktop (Windows, macOS, Linux) all from a single codebase.
- Fast & Smooth: Delivers a native look and feel on each platform with smooth performance.
- Visually Appealing: Rich set of widgets and customization options for stunning UIs.
- Developer Friendly: Easy to learn with features like hot reload for rapid iteration.
- Growing Community: Large and active community providing support and resources.
Base64 Encoding & Decoding in Dart & Flutter
Dart empowers developers with seamless Base64 encoding and decoding capabilities. The built-in dart:convert
library provides user-friendly functions like base64.encode
and base64.decode
that streamline the conversion of binary data into human-readable Base64 strings and vice versa. This simplifies working with Base64-encoded data within your Dart applications, making it easy to integrate and utilize this common encoding format.
To encode binary data into Base64 format, you can use the dart:convert
library’s base64.encode()
function. This function takes a list of bytes as input and returns the corresponding Base64-encoded string.
To decode a Base64-encoded string back to its original binary form, you can use the dart:convert
library’s base64.decode()
function. This function takes a Base64-encoded string as input and returns a list of bytes representing the original binary data.
Example of Base64 Encoding in Dart & Flutter
In this encoding example, the original text string is changed into a list of bytes using the utf8.encode()
function. The bytes are then encoded into a Base64-encoded string using the base64.encode()
function. Both the original text and the encoded text are shown in the output.
import 'dart:convert'; void main() { String originalText = "B64Encode"; List<int> bytes = utf8.encode(originalText); String encodedText = base64.encode(bytes); print("Original Text: $originalText"); print("Encoded Text: $encodedText"); }
Example of Base64 Decoding in Dart & Flutter
In this decoding example, we begin with a string that has been Base64 encoded. The encoded text is decoded using the base64.decode() method and returned as a list of bytes. We change the bytes into the original text string using utf8.decode(). Both the encoded and the decoded text are shown in the output.
import 'dart:convert'; void main() { String encodedText = "QjY0RW5jb2Rl"; List<int> bytes = base64.decode(encodedText); String decodedText = utf8.decode(bytes); print("Encoded Text: $encodedText"); print("Decoded Text: $decodedText"); }
Example Of Encoding An Image To Base64 (Uint8List To Base64)
In Flutter, encoding an image to Base64 entails reading the image file, turning it to an Uint8List (a list of bytes), and then encoding it to Base64 using the dart:convert library.
Here’s an example of how to encode a picture in Flutter to Base64:
import 'dart:convert'; import 'dart:io'; void main() { // Replace with the path to your image file String imagePath = 'path_to_your_image.png'; // Read the image file as bytes File imageFile = File(imagePath); Uint8List bytes = imageFile.readAsBytesSync(); // Encode the bytes to Base64 String base64EncodedImage = base64Encode(bytes); // Print the Base64-encoded image print("Base64 Encoded Image:\n$base64EncodedImage"); }
In this example:
- You specify the path to your image file in the
imagePath
variable. - The
File
class is used to read the image file as bytes. Make sure to replace'path_to_your_image.png'
with the actual path to your image file. - The
base64Encode()
function from thedart:convert
library is used to encode the image bytes into Base64 format. - The resulting Base64-encoded image is printed to the console.
Example Of Decoding Base64 To An Image
In Flutter, decoding Base64 data back into an image entails taking the Base64-encoded string, decoding it to a list of bytes, and then producing an image from those bytes.
Here’s an example of how to decode Base64 data into an image:
import 'dart:convert'; import 'dart:typed_data'; import 'dart:io'; void main() { // Replace with your Base64-encoded image data String base64EncodedImage = "Your_Base64_Encoded_Image_Data"; // Decode Base64 data to bytes Uint8List bytes = base64.decode(base64EncodedImage); // Create an image from the decoded bytes File imageFile = File('decoded_image.png'); // Output image file path imageFile.writeAsBytesSync(bytes); print("Image decoded and saved as ${imageFile.path}"); }
In this example:
- Replace
"Your_Base64_Encoded_Image_Data"
with the actual Base64-encoded image data that you want to decode. - The
base64.decode()
function from thedart:convert
library is used to decode the Base64-encoded string into aUint8List
(a list of bytes). - You create an image file (in this case, named
'decoded_image.png'
) to save the decoded image. You can specify the path and filename according to your needs. - Finally, the decoded image data is written to the image file using
imageFile.writeAsBytesSync(bytes)
.
After running this code, the decoded picture will be stored as the chosen file, which you can subsequently use or show as needed. Make sure to replace the placeholder "Your_Base64_Encoded_Image_Data"
with your actual Base64-encoded image data.
Example Of Converting PDF To Base64
Here’s an example of how to convert a PDF file to Base64 in Flutter.
import 'dart:convert'; import 'dart:io'; void main() { // Replace with the path to your PDF file String pdfPath = 'path_to_your_pdf.pdf'; // Read the PDF file as bytes File pdfFile = File(pdfPath); List<int> bytes = pdfFile.readAsBytesSync(); // Encode the bytes to Base64 String base64EncodedPDF = base64Encode(Uint8List.fromList(bytes)); // Print the Base64-encoded PDF print("Base64 Encoded PDF:\n$base64EncodedPDF"); }
In this example:
- You specify the path to your PDF file in the
pdfPath
variable. - The
File
class is used to read the PDF file as a list of bytes. Ensure that you replace'path_to_your_pdf.pdf'
with the actual path to your PDF file. - The
base64Encode()
function from thedart:convert
library is used to encode the PDF bytes into Base64 format. - The resulting Base64-encoded PDF is printed to the console.
Remember to replace the file path with the actual path to your PDF file, and ensure that the PDF file exists in the specified location.