In this article, we’ll explore techniques and tools for working with Base64 in ReactJS & Node.js, empowering you to create more efficient and secure web applications.
We’ll delve into built-in functions like btoa() and atob(), as well as the versatile Buffer class, to help you effectively encode and decode data in your ReactJS projects.
By understanding these concepts and tools, you can enhance your ability to work with images, files, and other data types, ultimately improving the performance and functionality of your ReactJS applications.
What is Base64?
Base64 is a popular binary-to-text encoding method in computing and data transmission. It enables binary data, such as images or files, to be represented by a set of printable ASCII letters, providing secure and reliable communication across several protocols. Base64 encoding separates binary data into six-bit groups, which are then translated to specified ASCII characters chosen for compatibility and security.
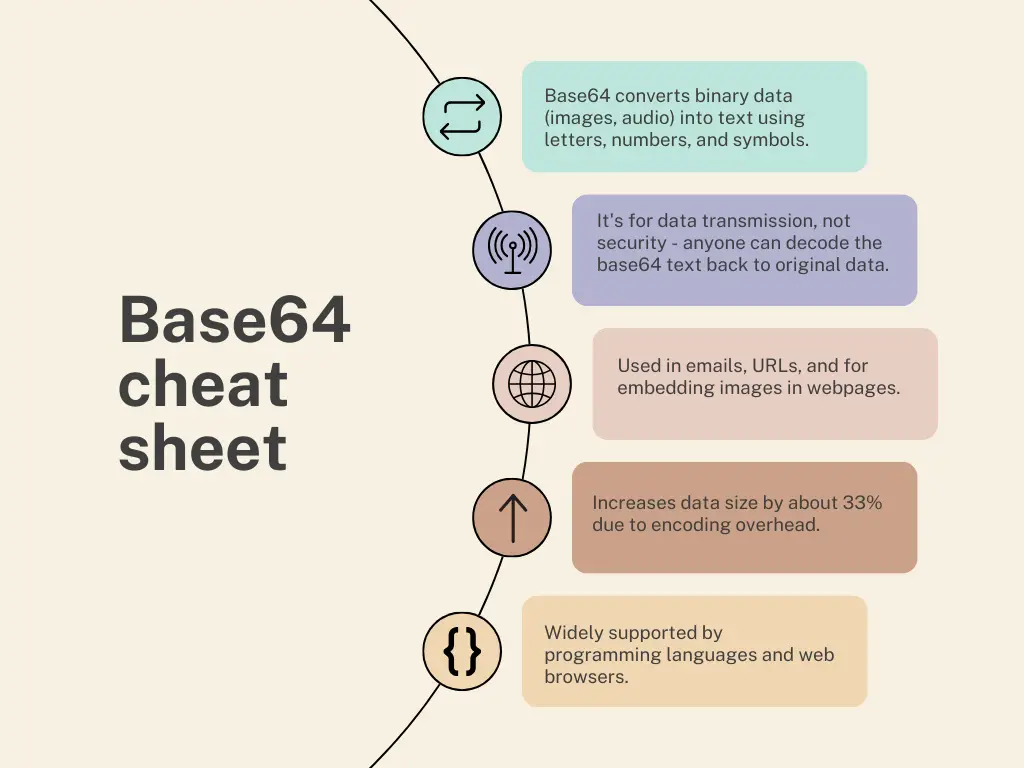
This encoding method is often used in situations when binary data must be conveyed in a text-based format. While Base64 encoding increases data size by around one-third when compared to its original binary form, it is nonetheless widely used due to its ubiquitous support in programming languages and its application in web development, data transfer, and other disciplines. It is important to note that Base64 encoding does not provide encryption in and of itself, but rather acts as a foundational tool for operations such as delivering binary data in URLs, embedding graphics, and more.
What is ReactJS?
ReactJS, commonly known as React or React.js, is a powerful and widely-used JavaScript library that has revolutionized the way developers create dynamic and interactive user interfaces for web applications. Developed and maintained by Facebook, React empowers developers to design reusable user interface components, ensuring efficient updates to the user interface as the application’s state changes. At the heart of React’s success lies its component-based architecture and virtual DOM implementation, which optimize performance and streamline the development process.
React adheres to a component-based architecture, in which large user interfaces are created by assembling smaller, modular components. This modular approach promotes code reusability, maintainability, and scalability, enabling developers to build complex applications with ease.
React’s virtual DOM (Document Object Model) implementation is one of its guiding principles. Instead of directly manipulating the DOM, React builds a virtual representation of the DOM in memory. When changes occur, this virtual DOM allows React to efficiently update and render only the appropriate components, reducing the performance cost associated with direct DOM manipulation. This innovative approach significantly improves rendering speed and enhances the overall user experience, making React an indispensable tool for modern web development.
A quick overview of ReactJS:
- Building Blocks Approach: Creates complex UIs by combining reusable components, making code more manageable.
- Declarative Style: Focuses on describing what the UI should look like, letting React handle the updates efficiently.
- Virtual DOM: Optimizes performance by minimizing actual DOM manipulations in the browser.
- Large Community & Ecosystem: Benefits from a vast community and rich ecosystem of tools and libraries.
- Suitable for SPAs & Web Apps: A great choice for building dynamic single-page applications and interactive web experiences.
Base64 Encoding and Decoding in ReactJS & Node.js
While working with ReactJS, you may encounter situations where you need to perform Base64 encoding and decoding. Although ReactJS does not offer native built-in functions for these operations, there are alternative solutions available. You can leverage JavaScript’s btoa()
and atob()
functions for basic Base64 operations, or use the Buffer
class of Node.js for more advanced encoding and decoding tasks.
JavaScript’s btoa() and atob() functions are simple and easy-to-use tools for basic Base64 encoding and decoding. These functions can be useful for quick, one-off encoding or decoding tasks within your ReactJS application. However, they may not be suitable for more complex operations or larger data sets.
For more advanced Base64 encoding and decoding tasks, you can turn to the Buffer class provided by Node.js. The Buffer class offers a wider range of functionality and is better equipped to handle larger data sets and more complex encoding and decoding operations. By harnessing the power of the Buffer class, you can efficiently manage Base64 operations within your ReactJS application, ensuring seamless data transfer and manipulation.
Example with btoa()
and atob()
In ReactJS, you can use JavaScript’s built-in btoa()
and atob()
functions to perform basic Base64 encoding and decoding operations. These functions are simple and easy to use, making them ideal for quick, one-off encoding or decoding tasks within your application. Here’s a simple demonstration of how you can use btoa() to encode a string to Base64, and atob() to decode a Base64 string back to its original form:
const originalText = 'B64Encode.com'; const encodedText = btoa(originalText); const decodedText = atob(encodedText); console.log(encodedText); console.log(decodedText);
Example with Buffer
in Node.js Environment
When working with React applications built using server-side rendering or other Node.js environments, the Buffer
class offers a powerful solution for more complex Base64 encoding and decoding tasks. The Buffer class provides a wider range of functionality compared to the btoa() and atob() functions, making it better suited for handling larger data sets and more advanced operations.
Here’s a look at how you can utilize the Buffer class to perform advanced Base64 encoding and decoding in your Node.js-based React applications:
// Import Buffer if running in a Node.js environment const Buffer = require('buffer').Buffer; const originalText = 'B64Encode.com'; const encodedBuffer = Buffer.from(originalText, 'utf-8').toString('base64'); const decodedBuffer = Buffer.from(encodedBuffer, 'base64').toString('utf-8'); console.log(encodedBuffer); console.log(decodedBuffer);
Keep in mind that the Buffer
class is available in Node.js environments, and the browser doesn’t support it natively. If you’re working within a browser environment, stick with the btoa()
and atob()
functions for Base64 encoding and decoding tasks.