In this post, we’ll look at how to utilize Base64 encoding in the MongoDB database. We’ll begin by defining Base64 and providing an overview of MongoDB’s primary features. After that, we’ll go over a real-world example of storing and saving Base64-encoded photos in MongoDB. Finally, we’ll look at the Base64 decoding procedure in the MongoDB context. Join us as we explore the potential of Base64 in MongoDB!
What is Base64?
Base64 is a binary-to-text encoding technique that is commonly used to encode binary data when it has to be preserved and moved across text-based medium. This encoding ensures that the data remains unmodified throughout transport. Base64 is extensively utilized in a broad range of applications, including email and the storage of complex data in XML or JSON.
The binary data is converted into a series of 8-bit bytes, which are then represented as 64 separate printable characters in Base64 encoding, hence the name ‘Base64’. The characters chosen for the 64 required for the base can vary between implementations. The main technique is to select 64 characters that are members of a subset shared by most encodings as well as printable. Because of this combination, it is unlikely that the data will be altered in transit via services such as email, which were previously not 8-bit clean. The Base64 index table contains A-Z, a-z, 0-9, +, and / for the first 62 values. The ‘+’ and ‘/’ characters are used to pad the encoded value at the conclusion.
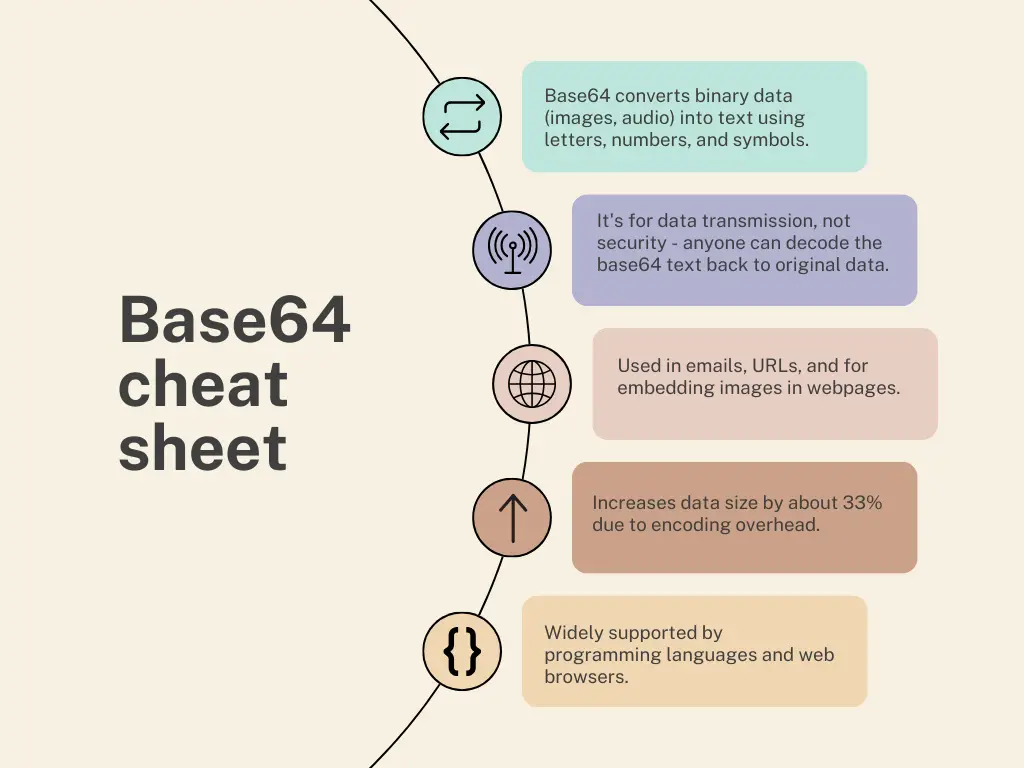
What is MongoDB?
MongoDB is a popular and capable NoSQL database management system that has grown in popularity in recent years. It is classified as a document-oriented database and is intended to manage enormous amounts of data with ease. MongoDB stands out for its adaptability, scalability, and aptitude for handling complex data structures with efficiency.
Key Characteristics of MongoDB:
- Document-Oriented: Unlike traditional relational databases that use tables and rows, MongoDB stores data in flexible, semi-structured BSON (Binary JSON) documents. This approach allows developers to work with data in a way that closely resembles the structure of their application code.
- Schema-less: MongoDB is schema-less, meaning that documents in a collection do not need to have the same structure. This flexibility enables developers to adapt to changing requirements without the need for complex migrations.
- Highly Scalable: MongoDB is designed to scale horizontally, making it well-suited for applications that need to handle increasing amounts of data and traffic. It can distribute data across multiple servers, ensuring high availability and fault tolerance.
- Rich Query Language: MongoDB provides a powerful query language that supports a wide range of queries, including geospatial queries, text searches, and more. It also supports indexing to optimize query performance.
- Aggregation Framework: MongoDB includes an aggregation framework that allows for complex data transformations and analytics, making it suitable for business intelligence and reporting applications.
- Community and Enterprise Versions: MongoDB offers both a free-to-use Community Edition and a paid Enterprise Edition. The Enterprise Edition includes additional features such as advanced security, monitoring, and support.
- Active Community and Ecosystem: MongoDB has a vibrant and active community of users and developers. It offers official drivers for multiple programming languages, making it accessible for developers across various technology stacks.
Use Cases for MongoDB:
- Content Management Systems (CMS): MongoDB can efficiently store and manage content in various formats, making it suitable for blogs, forums, and other content-driven websites.
- Real-Time Analytics: MongoDB’s aggregation framework and indexing capabilities make it a valuable tool for real-time analytics, allowing businesses to gain insights from their data quickly.
- IoT (Internet of Things): MongoDB can handle large volumes of data generated by IoT devices, making it a preferred choice for IoT applications that require real-time data processing and analysis.
- Catalogs and Product Management: E-commerce platforms often use MongoDB to manage product catalogs, as its schema-less nature accommodates the ever-changing product attributes.
- Mobile Applications: MongoDB’s support for geospatial queries and offline data synchronization makes it an excellent fit for mobile app development.
Example of Store/Save Base64 Image in MongoDB
When working with MongoDB, you can save images encoded in Base64 format directly into a database document.
Let’s explore a simple example of how to use Base64 encoding in MongoDB to store an image:
// Sample document TO represent an image var imageDocument = { _id: 1, imageName: "example.jpg", imageData: NEW Buffer.from("BinaryImageDataHere").toString("base64") }; // INSERT the document INTO a MongoDB collection db.images.insert(imageDocument);
In this example:
- We create a document with an
_id
,imageName
, andimageData
field. - The
imageData
field stores the binary image data as a Base64-encoded string. - We use the
Buffer.from
method to convert the binary image data into a buffer and then use.toString("base64")
to encode it as Base64.
Base64 Decoding in MongoDB
You can decode Base64-encoded data while obtaining data from MongoDB. The image data can be decoded as follows:
// Retrieve the image document var retrievedImage = db.images.findOne({_id: 1}); // Decode the Base64-encoded image DATA var decodedImageData = NEW Buffer.from(retrievedImage.imageData, "base64"); // Now, you can USE `decodedImageData` AS BINARY DATA
In this example, we retrieve the image document and then decode the imageData
field using the Buffer.from
method with the "base64"
encoding.